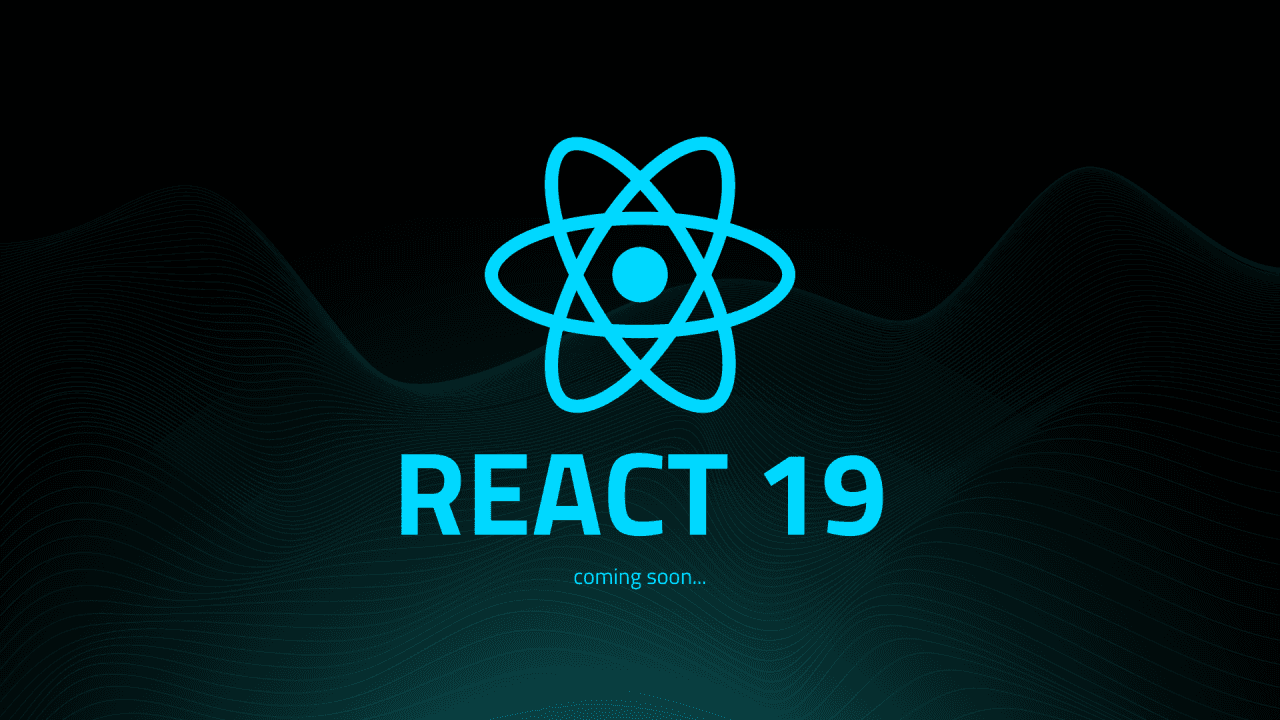
Technology
React 19 new features
By Dwinner ||
10 mins ||
4 July, 2024
React version 19 introduces a slew of groundbreaking features aimed at enhancing development efficiency and user experience. Here’s a sneak peek into what you can expect:
🔧 React Compiler: Already in use at Instagram, the React compiler promises streamlined development and optimized performance.
🌟 Server Components: Integrated with Next.js, server components offer efficient server-side rendering and improved performance.
💥 Actions: Redefining DOM interaction, Actions introduce a new paradigm for manipulating user interfaces.
📄 Document Metadata: Simplifies metadata management, reducing code while improving scalability and maintainability.
🚀 Assets Loading: Background loading of assets ensures faster app load times and smoother user experiences.
🔌 Web Components: Seamlessly integrates web standards into React applications, expanding compatibility.
🎣 Enhanced Hooks: Building on React’s success with hooks, version 19 introduces new hooks for cleaner, more maintainable code.
Maximizing Performance in React 19 with useMemo
Before:
import React, { useState, useMemo } from 'react';
function ExampleComponent() {
const [inputValue, setInputValue] = useState('');
// Memoize the result of checking if the input value is empty
const isInputEmpty = useMemo(() => {
console.log('Checking if input is empty...');
return inputValue.trim() === '';
}, [inputValue]);
return (
<div>
<input
type="text"
value={inputValue}
onChange={(e) => setInputValue(e.target.value)}
placeholder="Type something..."
/>
<p>{isInputEmpty ? 'Input is empty' : 'Input is not empty'}</p>
</div>
);
}
export default ExampleComponent;
After:
import React, { useState, useMemo } from 'react';
function ExampleComponent() {
const [inputValue, setInputValue] = useState('');
const isInputEmpty = () => {
console.log('Checking if input is empty...');
return inputValue.trim() === '';
});
return (
<div>
<input
type="text"
value={inputValue}
onChange={(e) => setInputValue(e.target.value)}
placeholder="Type something..."
/>
<p>{isInputEmpty ? 'Input is empty' : 'Input is not empty'}</p>
</div>
);
}
export default ExampleComponent;
Introducing the New use() Hook in React 19
React 19 is set to revolutionize state management and asynchronous operations with its innovative use() hook. This versatile hook simplifies the handling of promises, async code, and context within functional components, offering a more streamlined approach to modern web development.
Syntax:
const value = use(resource);
Here's a generated example demonstrating how to use the use() hook in React 19 to handle a fetch request:
import { use } from "react";
const fetchUsers = async () => {
const res = await fetch('https://example.com/users');
return res.json();
};
const UsersItems = () => {
const users = use(fetchUsers());
return (
<ul>
{users.map((user) => (
<div key={user.id}>
<h2>{user.name}</h2>
<p>{user.email}</p>
</div>
))}
</ul>
);
};
export default UsersItems;
The useOptimistic() hook
Introducing the useOptimistic() Hook in React
According to the React documentation, useOptimistic() is a powerful React Hook designed to display an optimistic state while an asynchronous action is in progress. This capability aims to enhance user experience by providing immediate feedback and potentially faster responses from the application, especially in scenarios where real-time interaction with a server is crucial.
Syntax:
const [ optimisticMessage, addOptimisticMessage] =
useOptimistic(state, updatefn)
Here's a generated example demonstrating how to implement the useOptimistic hook in React to show an optimistic state while awaiting a server response:
import { useOptimistic, useState } from "react";
const Optimistic = () => {
const [messages, setMessages] = useState([
{ text: "Hey, I am initial!", sending: false, key: 1 },
]);
const [optimisticMessages, addOptimisticMessage] = useOptimistic(
messages,
(state, newMessage) => [
...state,
{
text: newMessage,
sending: true,
},
]
);
async function sendFormData(formData) {
const sentMessage = await fakeDelayAction(formData.get("message"));
setMessages((messages) => [...messages, { text: sentMessage }]);
}
async function fakeDelayAction(message) {
await new Promise((res) => setTimeout(res, 1000));
return message;
}
const submitData = async (userData) => {
addOptimisticMessage(userData.get("username"));
await sendFormData(userData);
};
return (
<>
{optimisticMessages.map((message, index) => (
<div key={index}>
{message.text}
{!!message.sending && <small> (Sending...)</small>}
</div>
))}
<form action={submitData}>
<h1>OptimisticState Hook</h1>
<div>
<label>Username</label>
<input type="text" name="username" />
</div>
<button type="submit">Submit</button>
</form>
</>
);
};
export default Optimistic;
- fakeDelayAction is a fake method to delay the submit event. This is to show the optimistic state.
- submitData is the action. This method is responsible for the form submission. This could be async, too.
- sendFormData is responsible for sending the form to fakeDelayAction
- Setting the default state. messages will be used in the useOptimistic() as input and will return in optimisticMessages.
To keep abreast of the latest developments and updates from the React team, consider following them on the following platforms:
react
web
technology
Featured Post
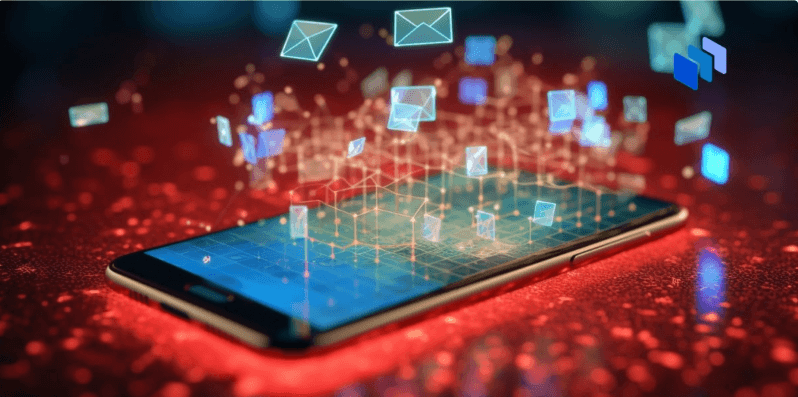
Tech SIM Swap Attacks: Analysis and Protective Mea...
Tech SIM Swap Attacks: Analysis and Protective Measures...
20 June, 2024
10 mins
Recent Post
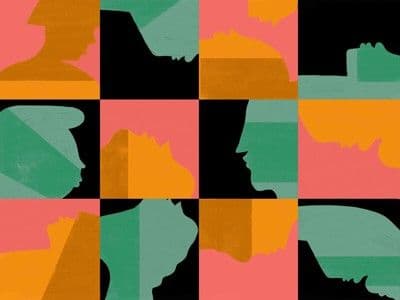
Computer science has a racism problem: these resea...
Black and Hispanic people face huge hurdles at technology companies an...
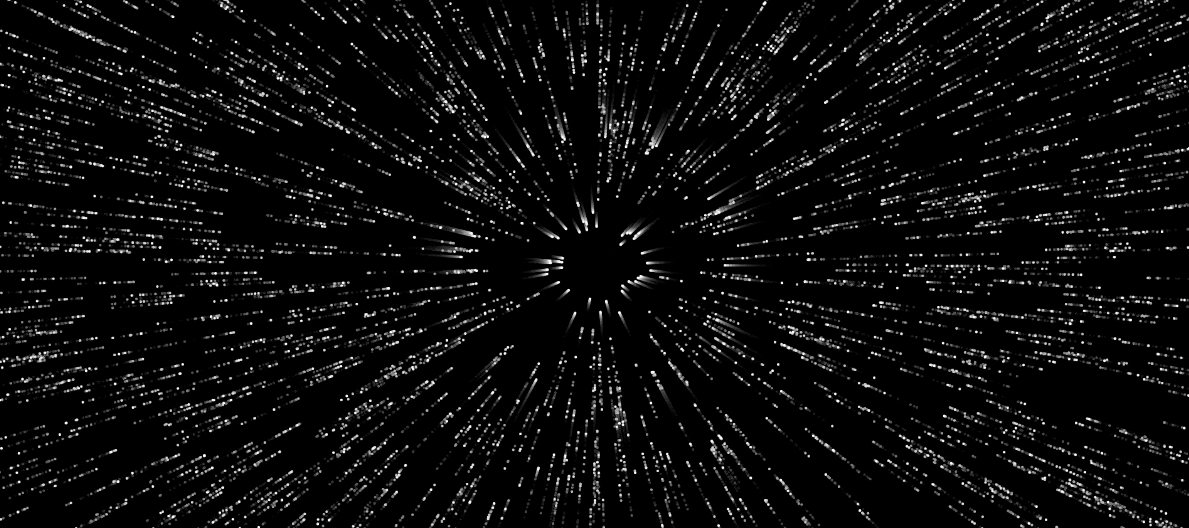
How to Generate Astonishing Animations with ChatGP...
How to Generate Astonishing Animations with ChatGPT
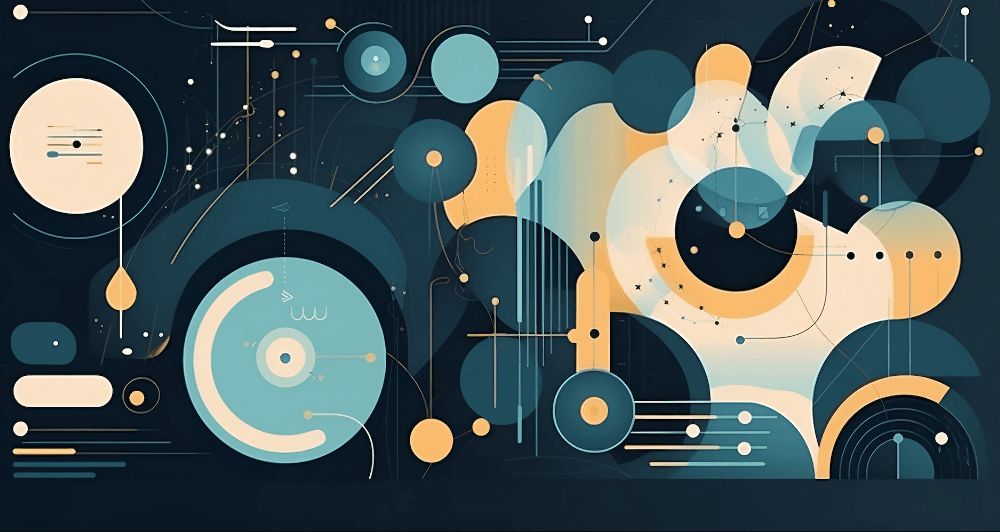
The Web’s Next Transition
The web is made up of technologies that got their start over 25 years ...
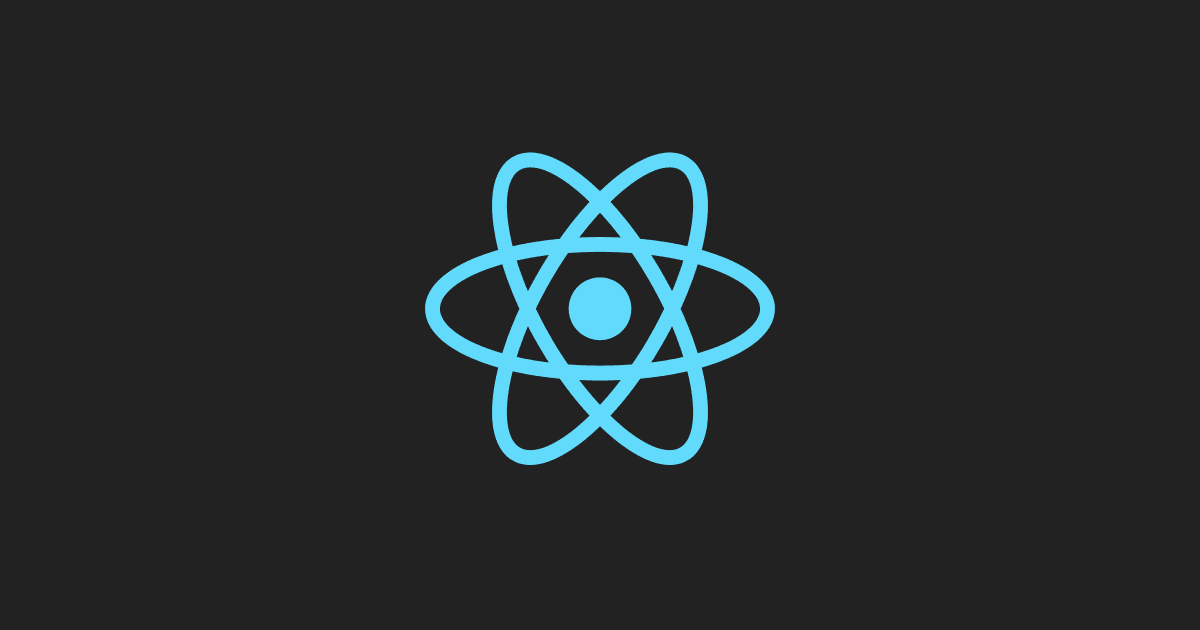
Fundamentals of React Hooks
Hooks have been really gaining a lot of popularity from the first day ...
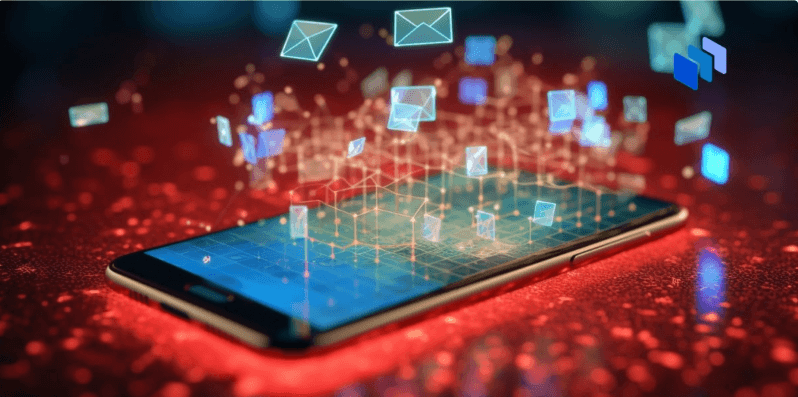
Tech SIM Swap Attacks: Analysis and Protective Mea...
Tech SIM Swap Attacks: Analysis and Protective Measures
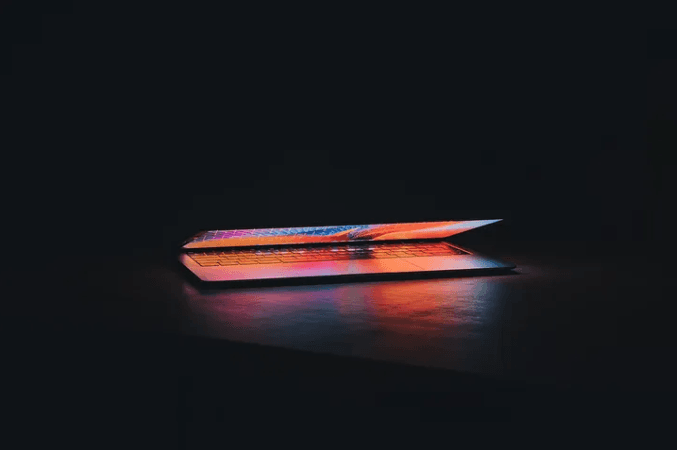
14 amazing macOS tips & tricks to power up your pr...
The mysteriOS Mac-hines keep having more hidden features the more you ...
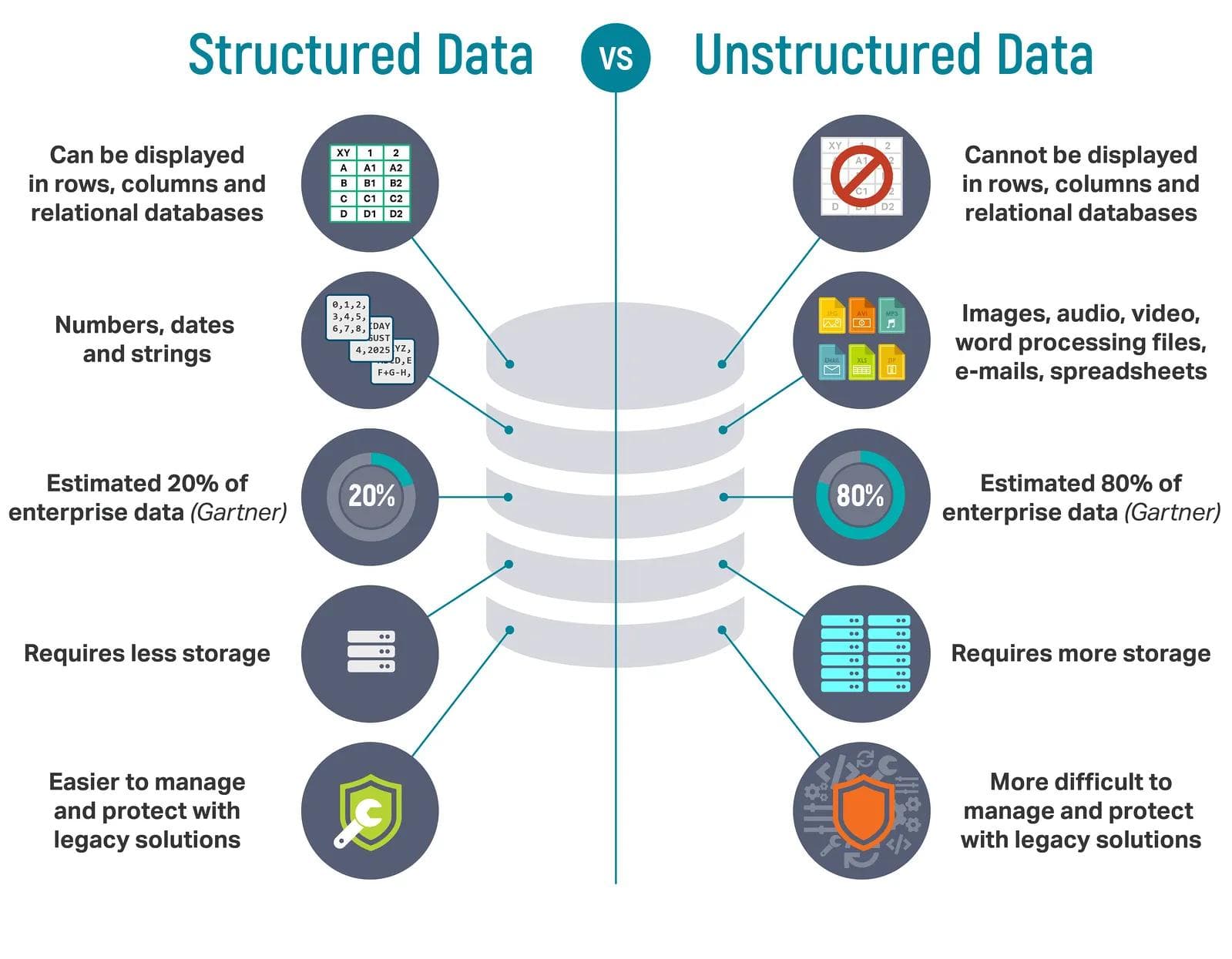
4 Easy Steps to Structure Highly Unstructured Big ...
4 Easy Steps to Structure Highly Unstructured Big Data, via Automated ...
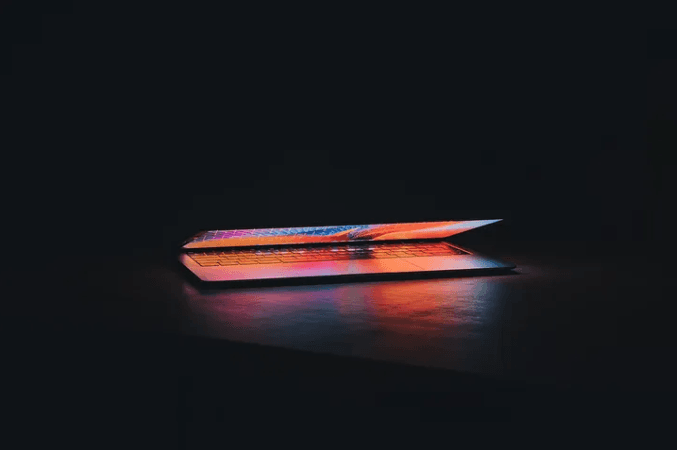
14 amazing macOS tips & tricks to power up your pr...
The mysteriOS Mac-hines keep having more hidden features the more you ...
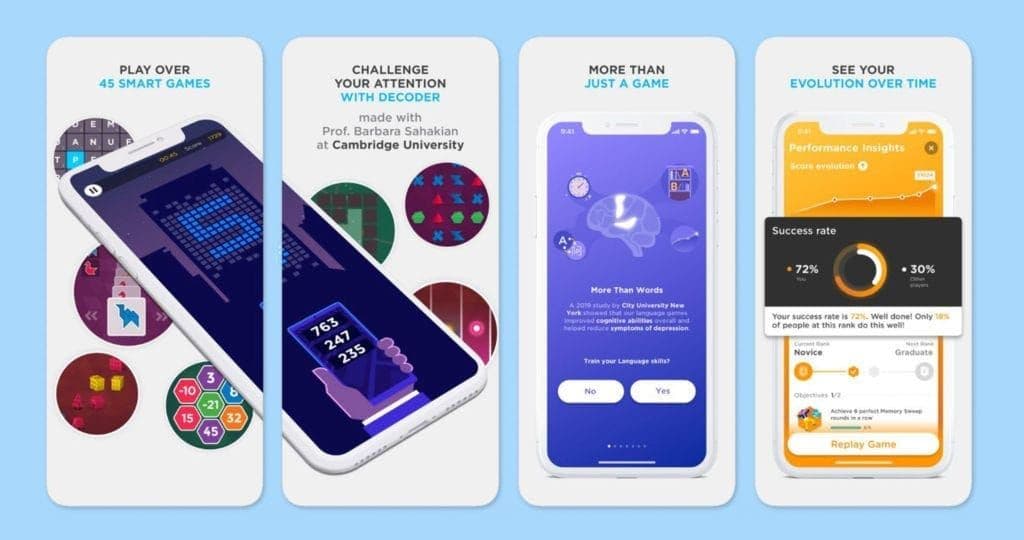
Top 20 mobile apps which nobody knows about…
In the vast ocean of mobile applications, some remarkable gems often g...
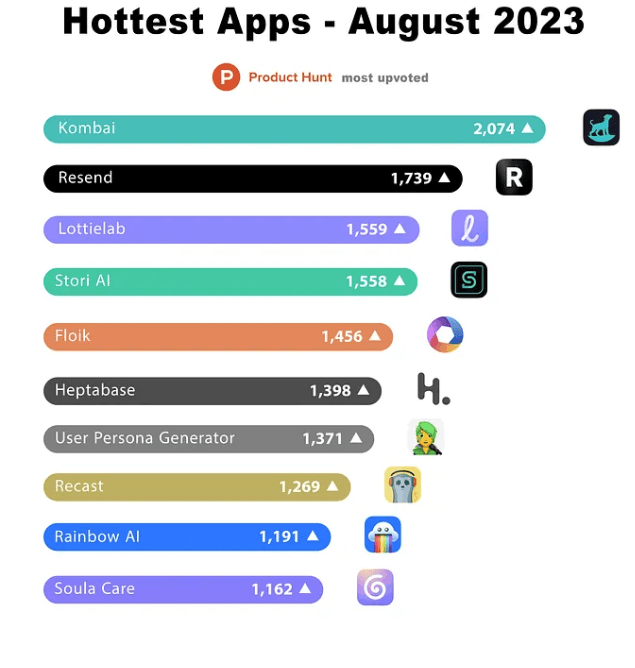
10 Hottest New Apps for You to Get Ahead in Septem...
Here we are again, in a tribute to the amazing stream of ideas that pr...
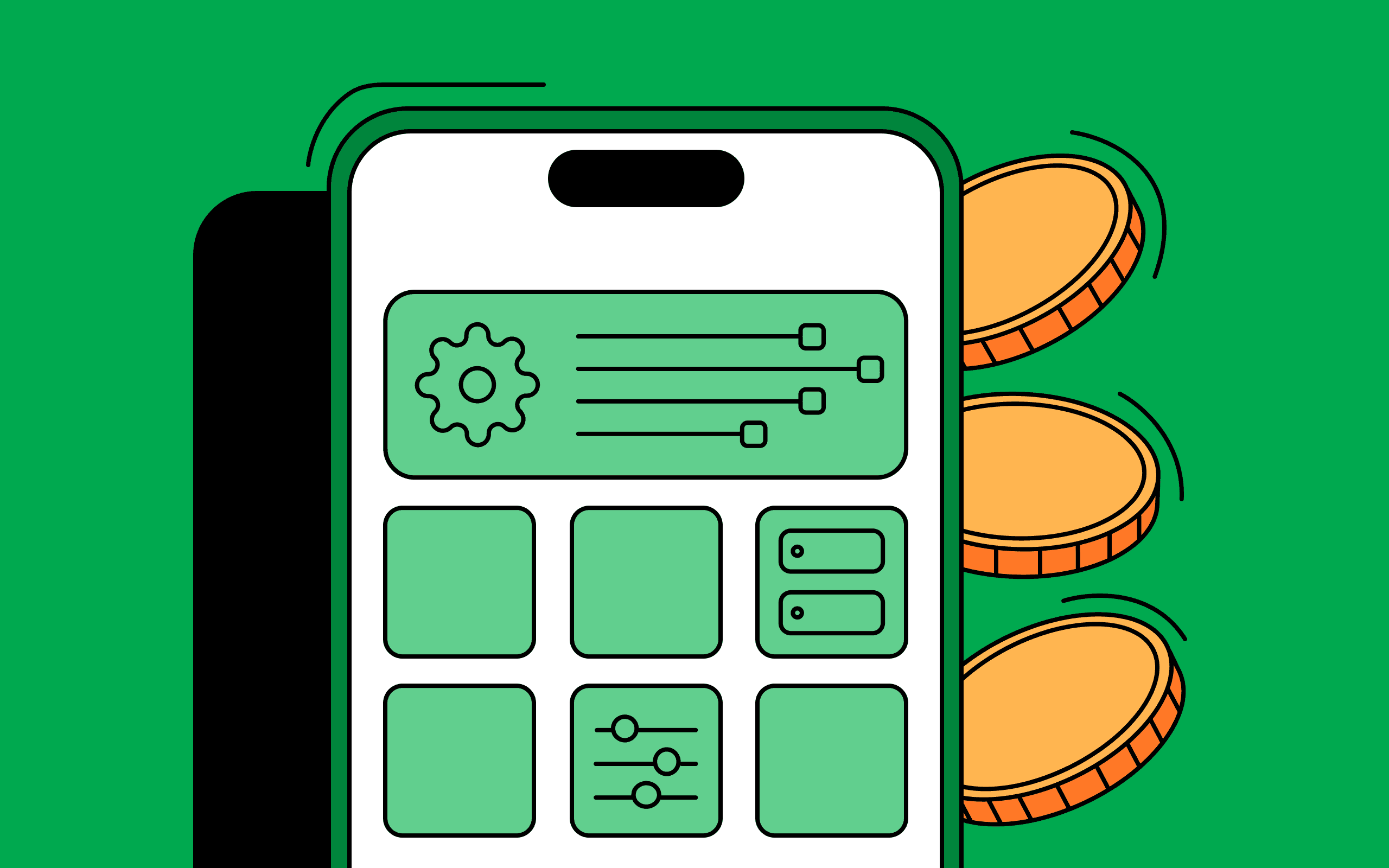
How much does mobile app development cost
The cost of developing a mobile application can vary depending on seve...
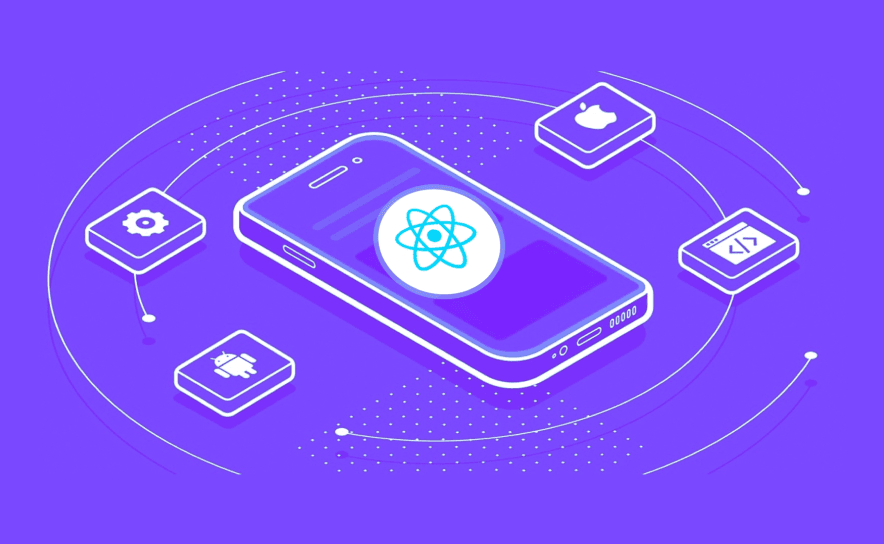
React Native App Development Guide
DWIN, founded in 2015, has swiftly established itself as a leader in c...
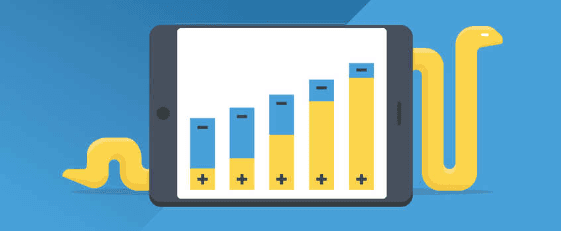
Comparison of Top 6 Python NLP Libraries
Comparison of Top 6 Python NLP Libraries
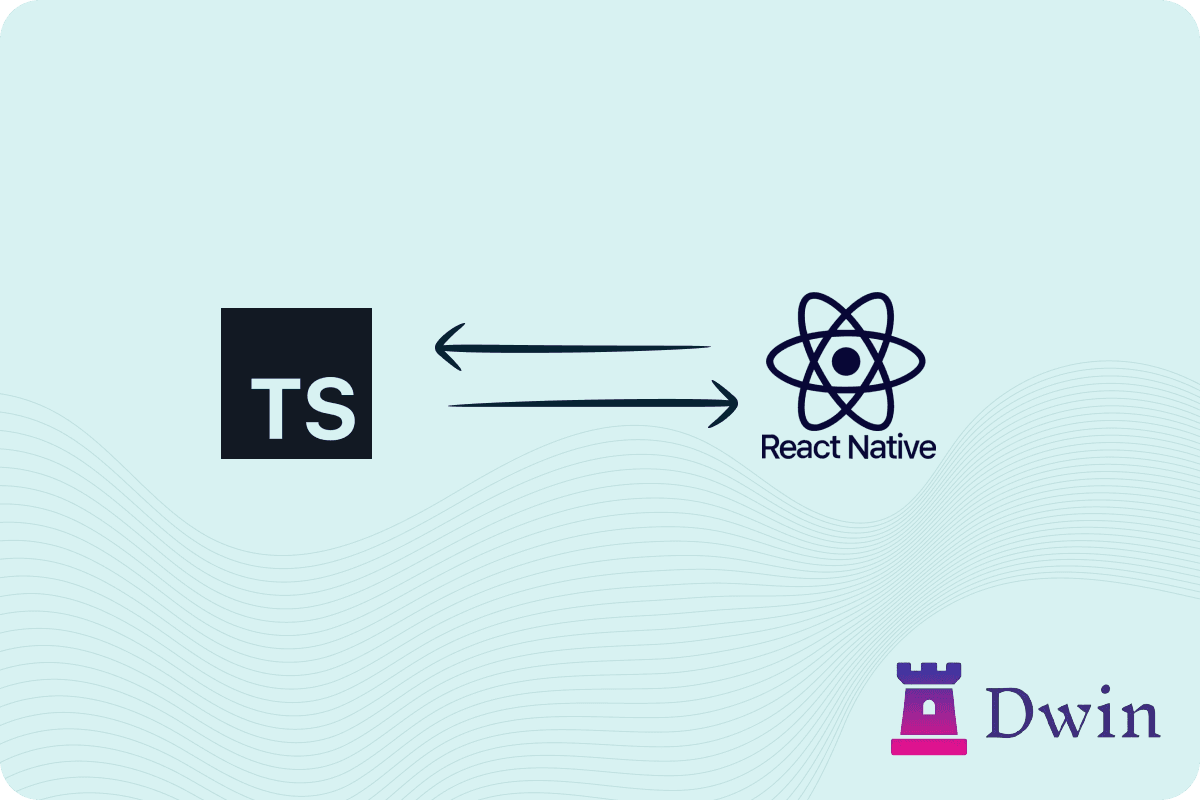
Type Checking with TypeScript in React Native Navi...
📱 In the realm of mobile app development with React Native, ensuring ...
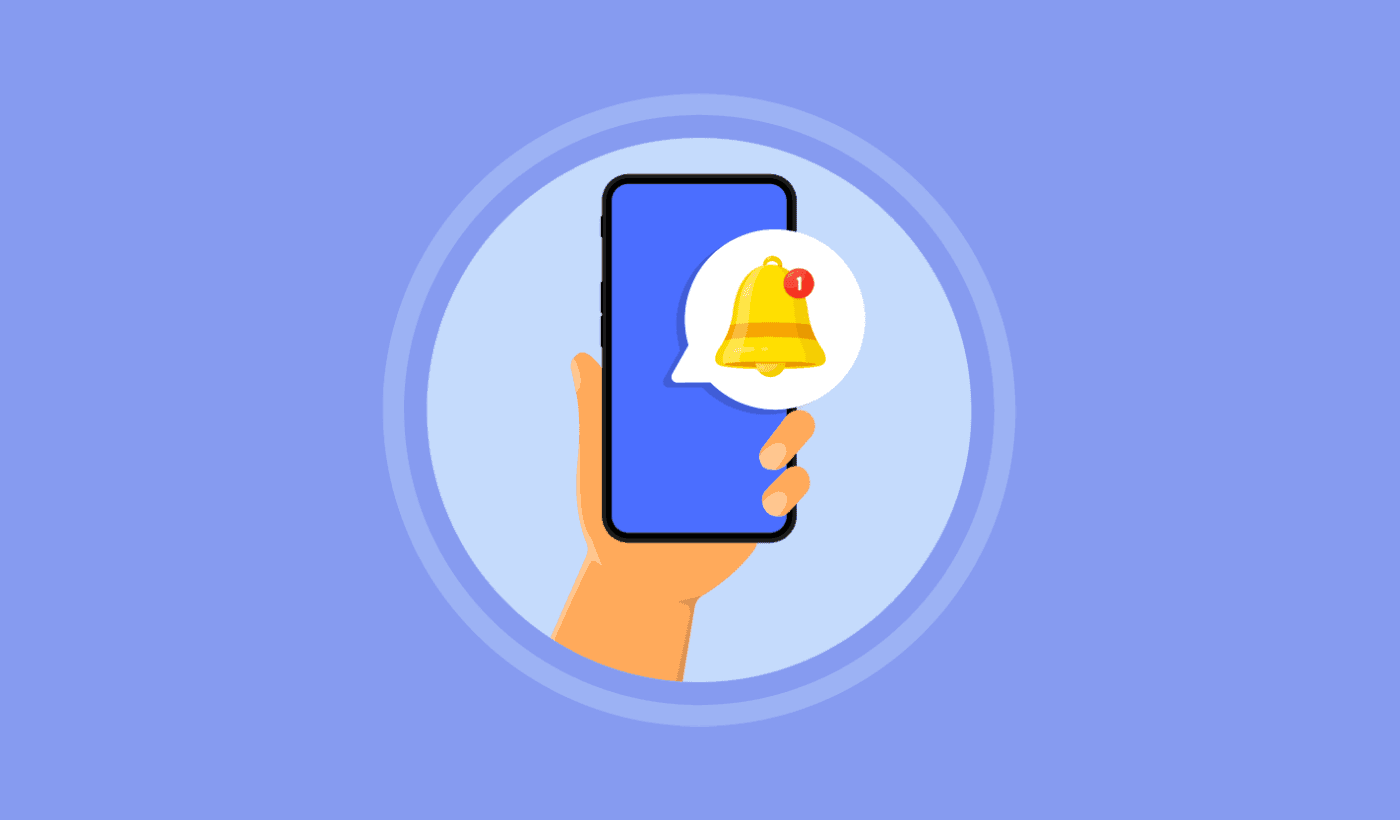
Guide to Set Up Push Notifications in React Native...
1. Create a New React Native Project (if not already created)
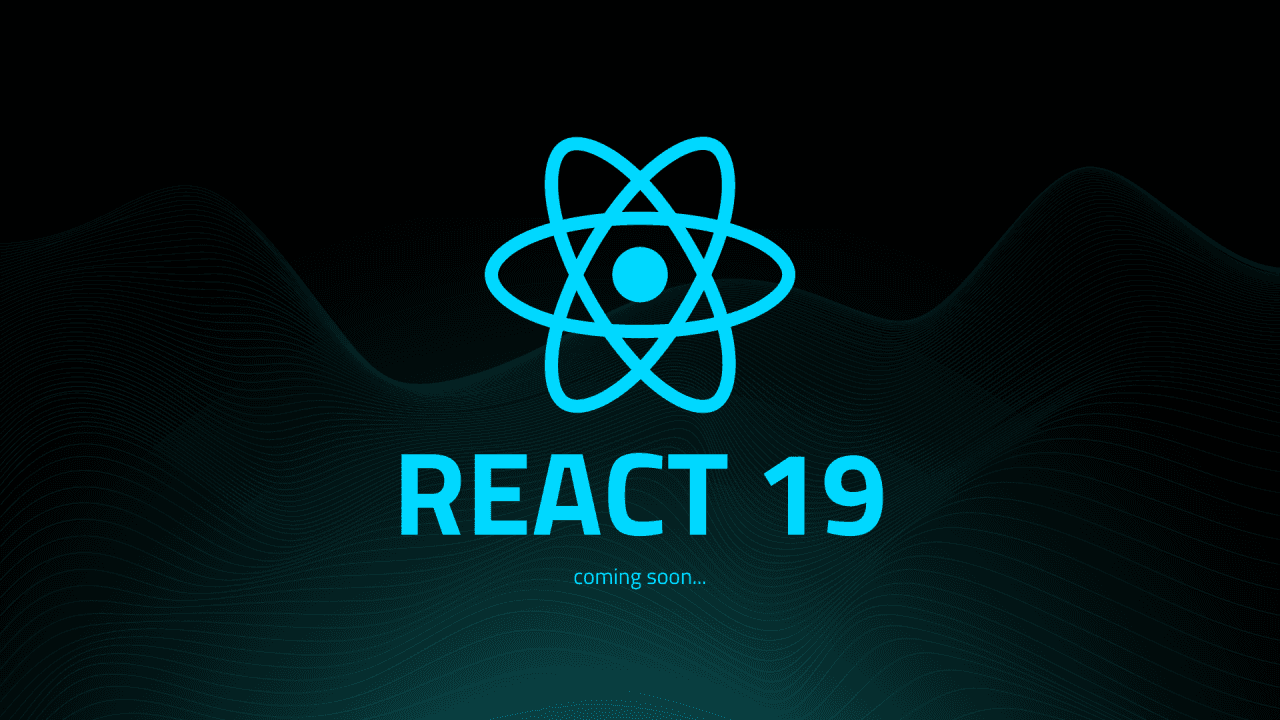
React 19 new features
React version 19 introduces a slew of groundbreaking features aimed at...