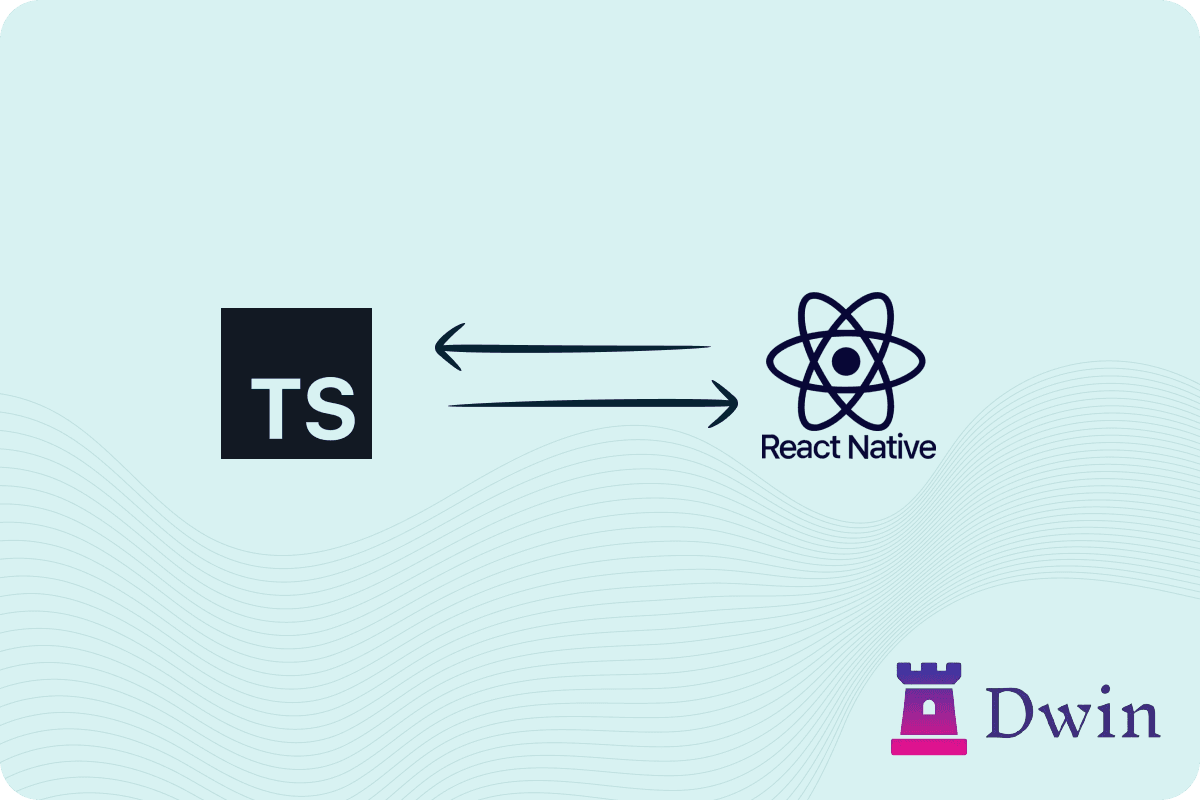
Technology
Type Checking with TypeScript in React Native Navigation
By Dwinner ||
ย 5 mins ||
ย 2 July, 2024
๐ฑ In the realm of mobile app development with React Native, ensuring code reliability and maintainability is paramount. TypeScript, a statically typed superset of JavaScript, proves invaluable in achieving these goals by providing robust type checking capabilities. Letโs explore how TypeScript can be effectively employed in a React Native navigation project, focusing on the following files within our navigation folder: index.tsx, navigationEnums.ts, and navigation.types.ts.
Understanding the Project Structure
1. index.tsx: ๐ This file serves as the main entry point or component for our navigation system in React Native.
2. navigationEnums.ts: ๐ Contains TypeScript enums defining constants related to navigation, ensuring clarity and consistency in navigation types.
3. navigation.types.ts: ๐๏ธ Houses TypeScript interfaces defining the structure of navigation-related objects, enhancing code documentation and maintainability.
Implementing TypeScript in Your React Native Navigation Project ๐
Let's delve into how TypeScript can be integrated across these files to leverage its powerful type checking capabilities effectively.
navigationEnums.ts ๐
Enums in TypeScript are ideal for defining a set of named constants, providing descriptive names to numeric values and improving code readability.
// navigationEnums.ts
export enum NavigationType {
Home = 'HOME',
About = 'ABOUT',
Contact = 'CONTACT',
// Add more navigation types as needed
}
Interfaces in TypeScript define the shape of objects, ensuring that objects conform to a specific structure and reducing errors in your React Native app. ๐
// navigation.types.ts
import { NavigationType } from './navigationEnums';
export interface NavigationItem {
title: string;
path: string;
type: NavigationType;
}
export interface NavigationState {
items: NavigationItem[];
selected: NavigationType | null;
}
Integrating TypeScript types and enums in your React Native navigation component ensures type safety and clarity throughout the application. ๐ก๏ธ
// index.tsx
import React, { useState } from 'react';
import { NavigationItem, NavigationType } from './navigation.types';
import { NavigationType as NavigationTypeEnum } from './navigationEnums';
const navigationItems: NavigationItem[] = [
{ title: 'Home', path: '/', type: NavigationTypeEnum.Home },
{ title: 'About', path: '/about', type: NavigationTypeEnum.About },
{ title: 'Contact', path: '/contact', type: NavigationTypeEnum.Contact },
// Add more navigation items as needed
];
const initialState = {
items: navigationItems,
selected: null as NavigationType | null,
};
const NavigationComponent: React.FC = () => {
const [state, setState] = useState(initialState);
const handleItemClick = (itemType: NavigationType) => {
setState(prevState => ({
...prevState,
selected: itemType,
}));
};
return (
<View>
<Text>๐ My Navigation</Text>
<FlatList
data={state.items}
keyExtractor={item => item.type}
renderItem={({ item }) => (
<TouchableOpacity onPress={() => handleItemClick(item.type)}>
<Text>{item.title}</Text>
</TouchableOpacity>
)}
/>
</View>
);
};
export default NavigationComponent;
Using TypeScript Types in Screen Components ๐
By incorporating TypeScript types in screen components, you ensure that data flowing through your React Native application adheres to the defined structure, reducing bugs and enhancing code maintainability.
// Example screen component using NavigationItem type
import React from 'react';
import { NavigationItem } from './navigation.types';
const Sidebar: React.FC<{ items: NavigationItem[] }> = ({ items }) => (
<View>
{items.map(item => (
<TouchableOpacity key={item.type} onPress={() => navigateTo(item.path)}>
<Text>๐ฒ {item.title}</Text>
</TouchableOpacity>
))}
</View>
);
export default Sidebar;
// Example screen component using NavigationItem type
import React from 'react';
import { NavigationItem } from './navigation.types';
const Sidebar: React.FC<{ items: NavigationItem[] }> = ({ items }) => (
<View>
{items.map(item => (
<TouchableOpacity key={item.type} onPress={() => navigateTo(item.path)}>
<Text>๐ฒ {item.title}</Text>
</TouchableOpacity>
))}
</View>
);
export default Sidebar;
Conclusion ๐
Incorporating TypeScript's strong typing features, such as enums and interfaces, into your React Native navigation project significantly enhances code clarity, maintainability, and reliability. TypeScript not only catches potential errors at compile-time but also improves code documentation and developer productivity. Embrace TypeScript in your React Native projects to build robust and scalable mobile applications with confidence.
Implement these strategies in your own React Native navigation project to experience firsthand the benefits of type checking with TypeScript. Happy coding! ๐
react-native
mobile
technology
mobile app development
Featured Post
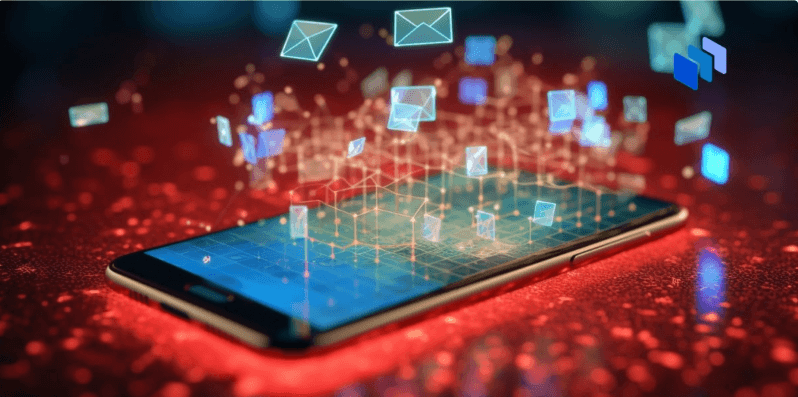
Tech SIM Swap Attacks: Analysis and Protective Mea...
Tech SIM Swap Attacks: Analysis and Protective Measures...
20 June, 2024
10 mins
Recent Post
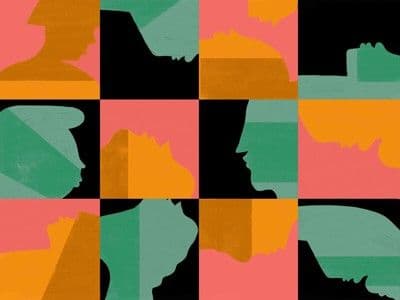
Computer science has a racism problem: these resea...
Black and Hispanic people face huge hurdles at technology companies an...
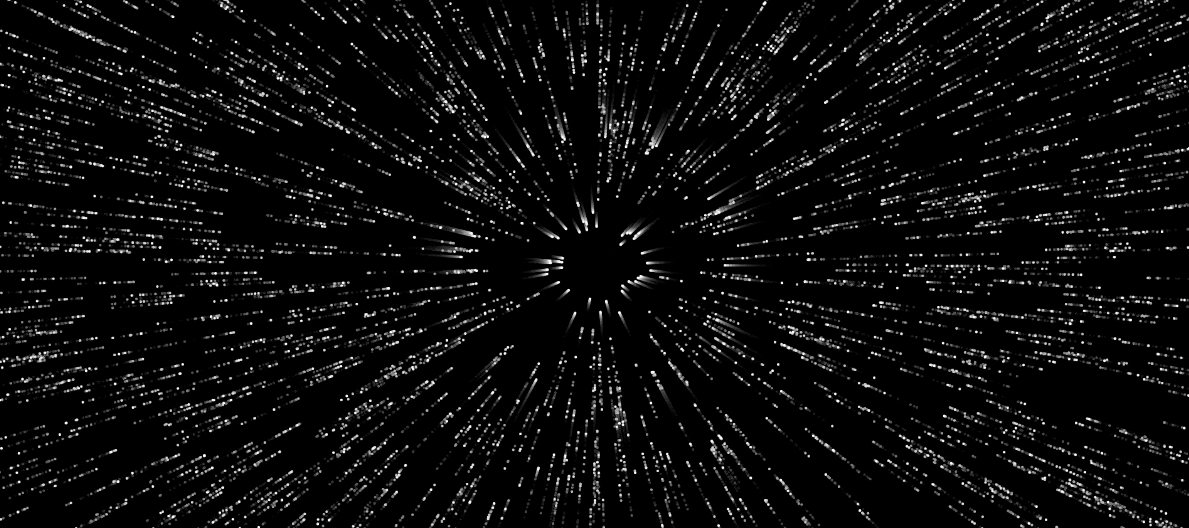
How to Generate Astonishing Animations with ChatGP...
How to Generate Astonishing Animations with ChatGPT
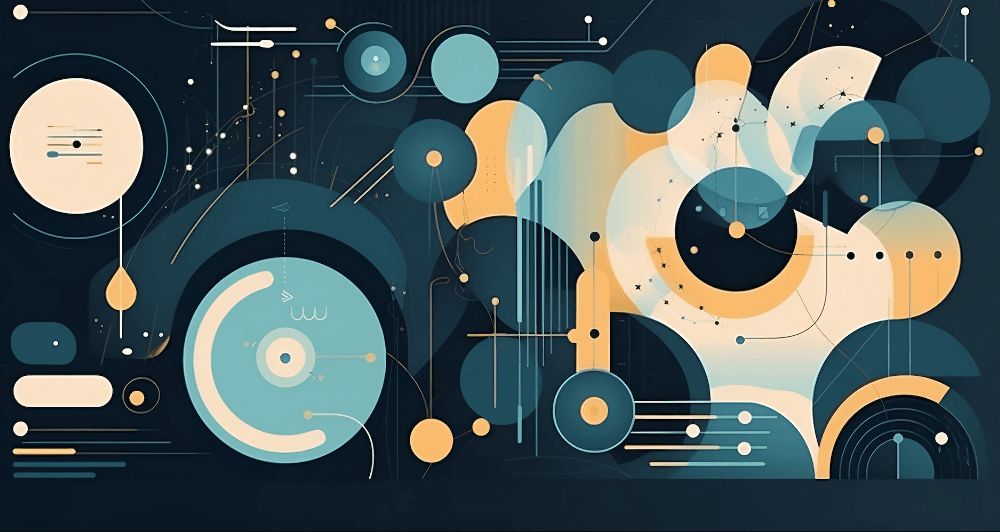
The Webโs Next Transition
The web is made up of technologies that got their start over 25 years ...
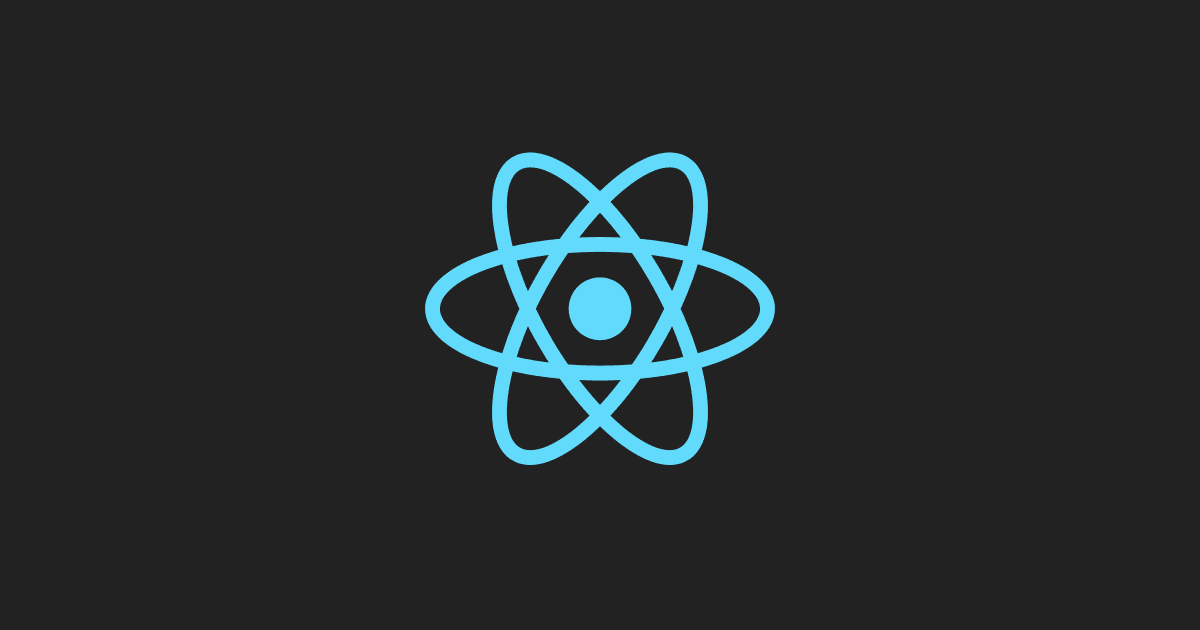
Fundamentals of React Hooks
Hooks have been really gaining a lot of popularity from the first day ...
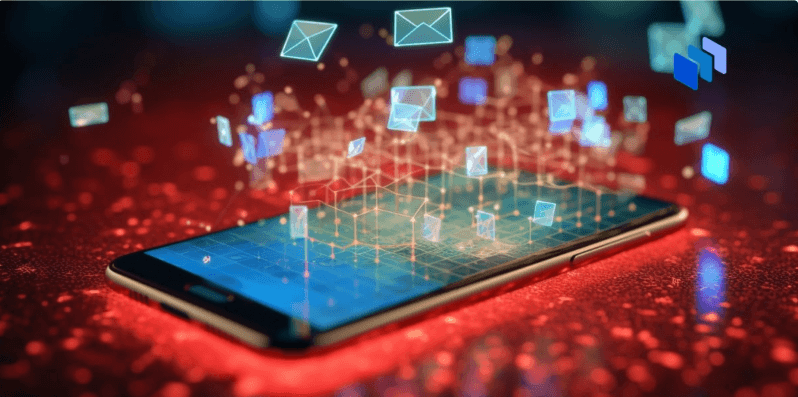
Tech SIM Swap Attacks: Analysis and Protective Mea...
Tech SIM Swap Attacks: Analysis and Protective Measures
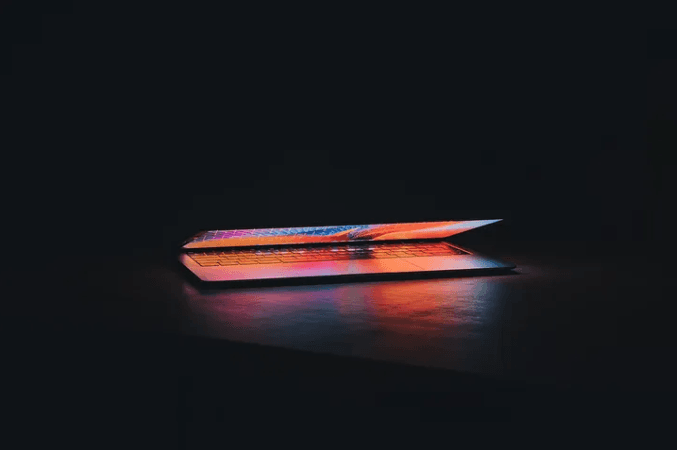
14 amazing macOS tips & tricks to power up your pr...
The mysteriOS Mac-hines keep having more hidden features the more you ...
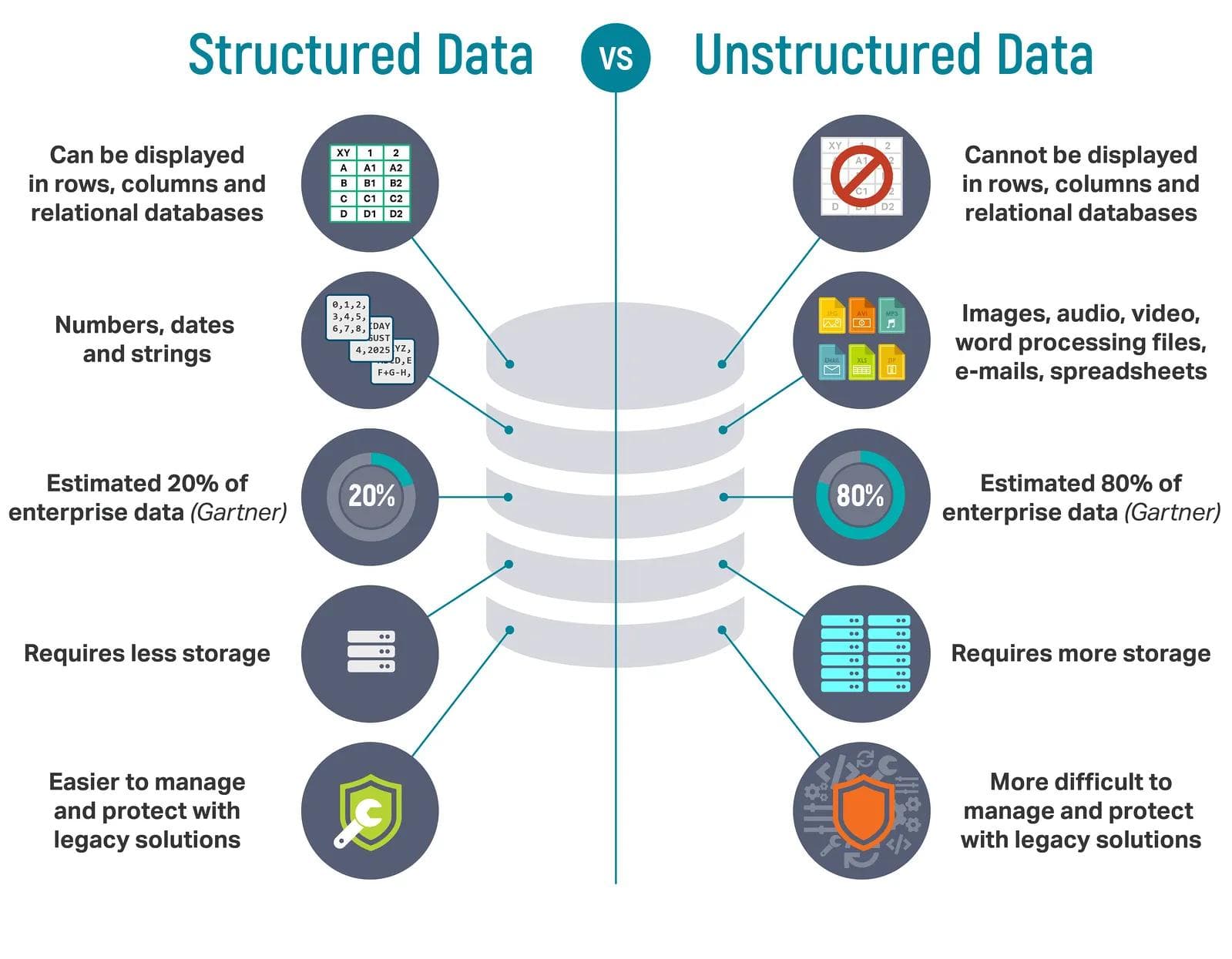
4 Easy Steps to Structure Highly Unstructured Big ...
4 Easy Steps to Structure Highly Unstructured Big Data, via Automated ...
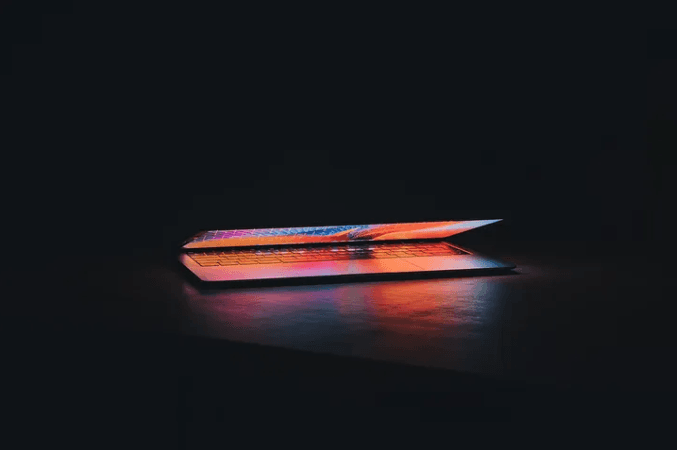
14 amazing macOS tips & tricks to power up your pr...
The mysteriOS Mac-hines keep having more hidden features the more you ...
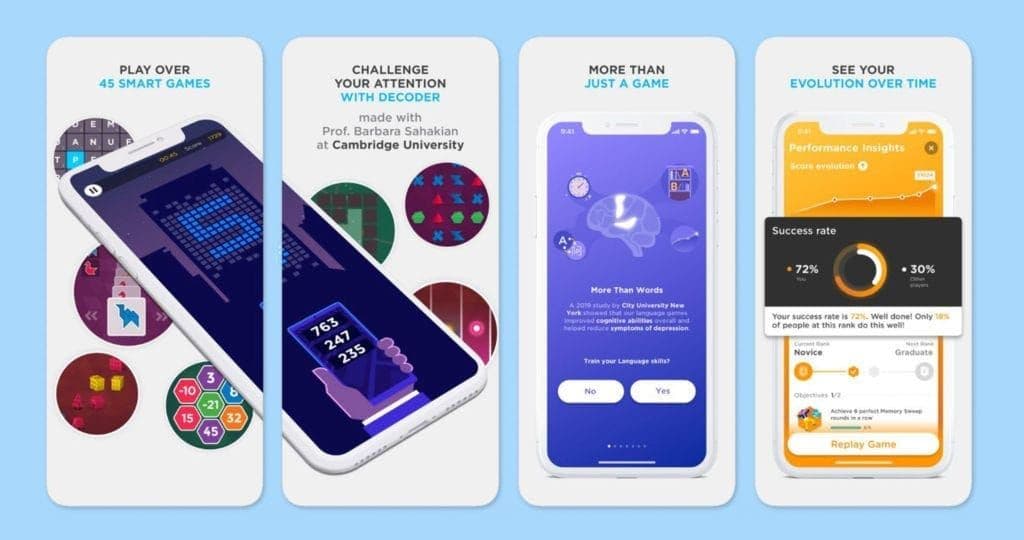
Top 20 mobile apps which nobody knows aboutโฆ
In the vast ocean of mobile applications, some remarkable gems often g...
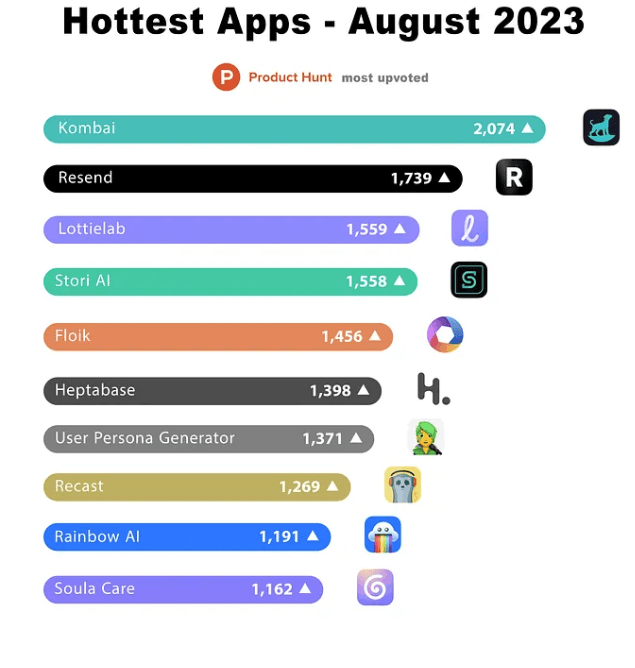
10 Hottest New Apps for You to Get Ahead in Septem...
Here we are again, in a tribute to the amazing stream of ideas that pr...
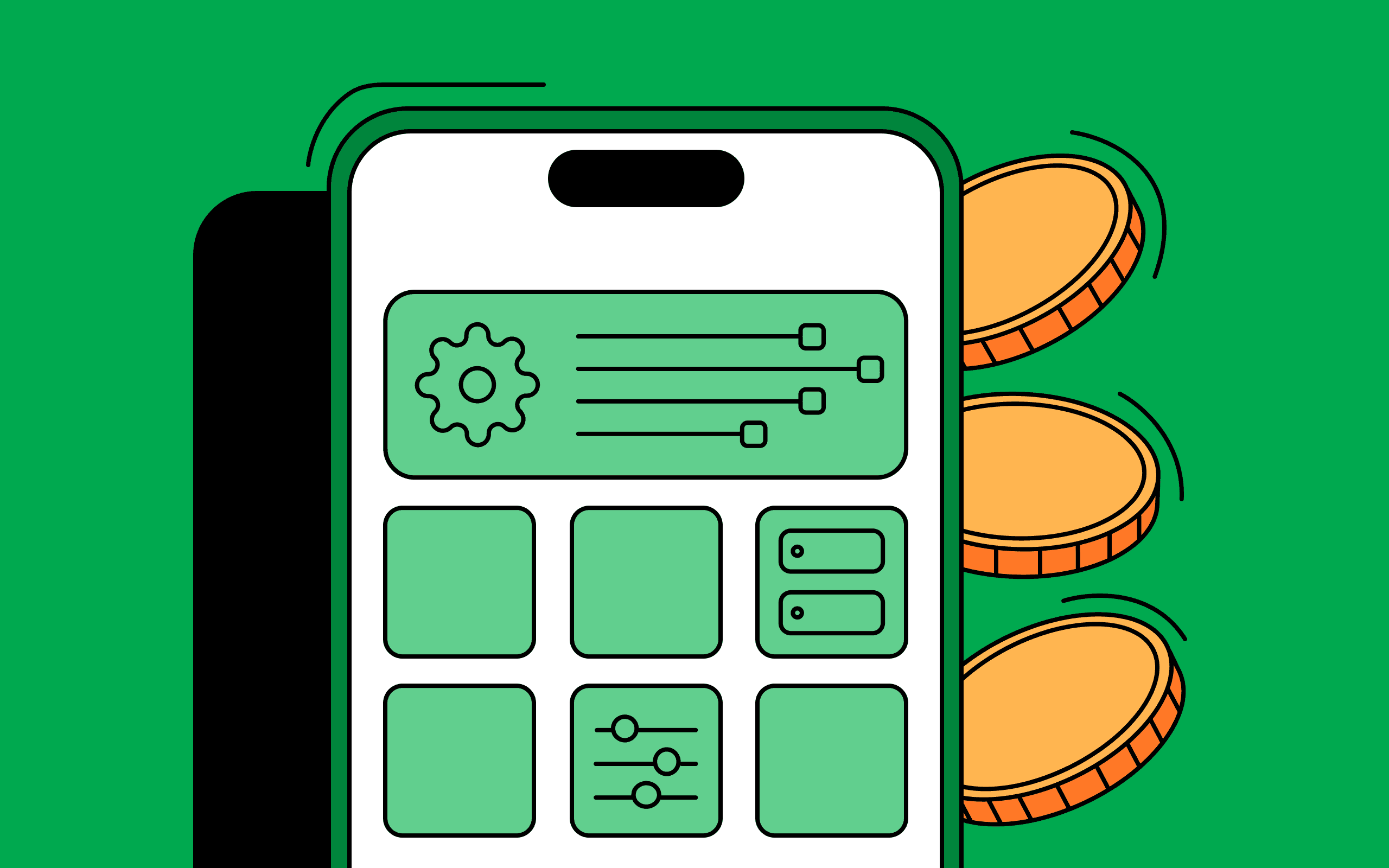
How much does mobile app development cost
The cost of developing a mobile application can vary depending on seve...
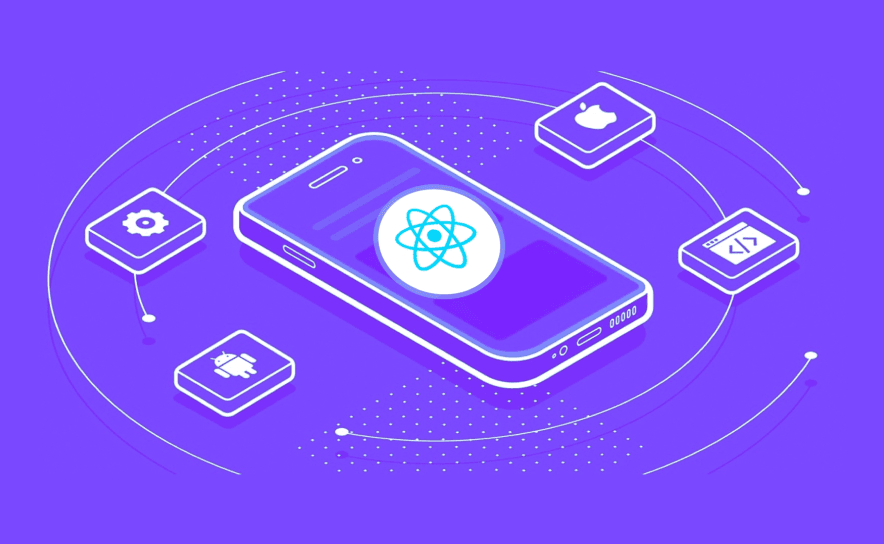
React Native App Development Guide
DWIN, founded in 2015, has swiftly established itself as a leader in c...
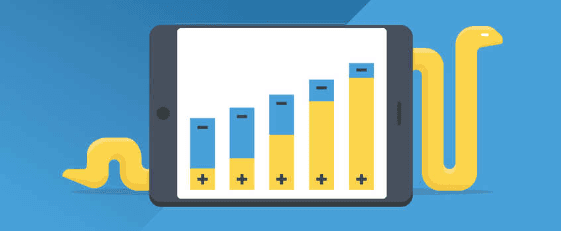
Comparison of Top 6 Python NLP Libraries
Comparison of Top 6 Python NLP Libraries
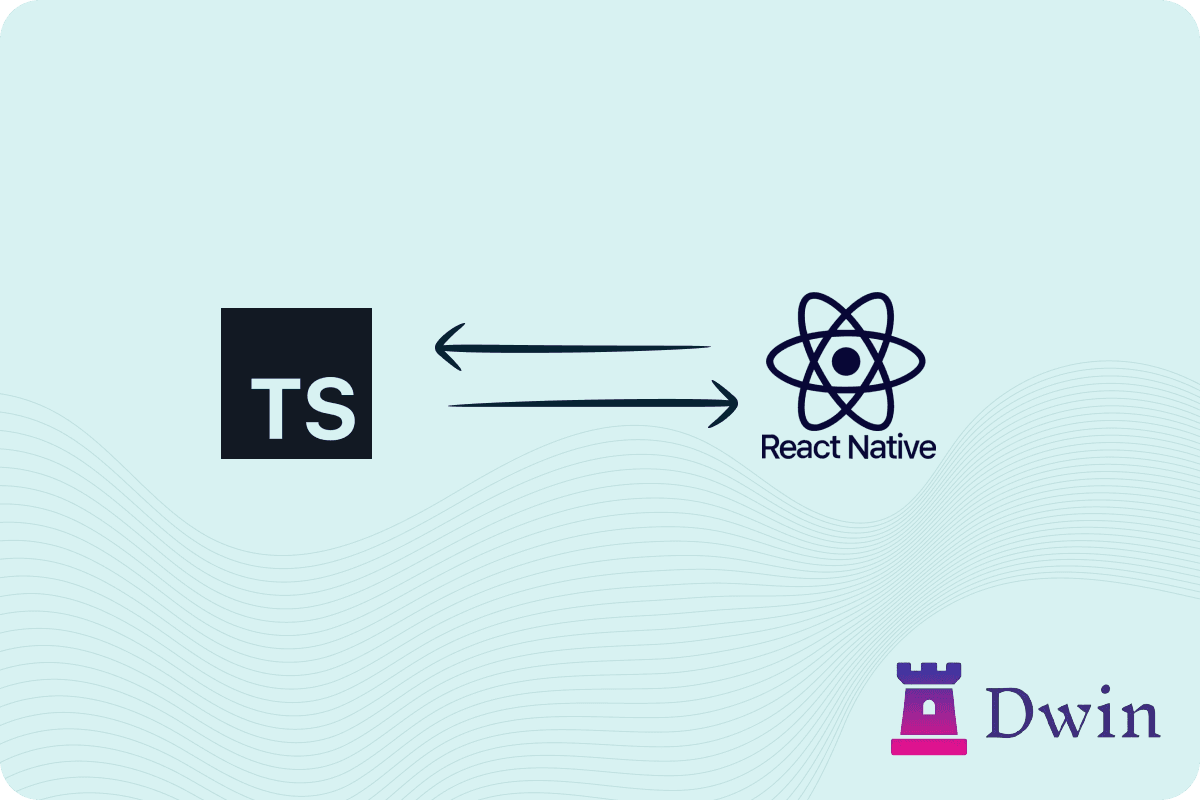
Type Checking with TypeScript in React Native Navi...
๐ฑ In the realm of mobile app development with React Native, ensuring ...
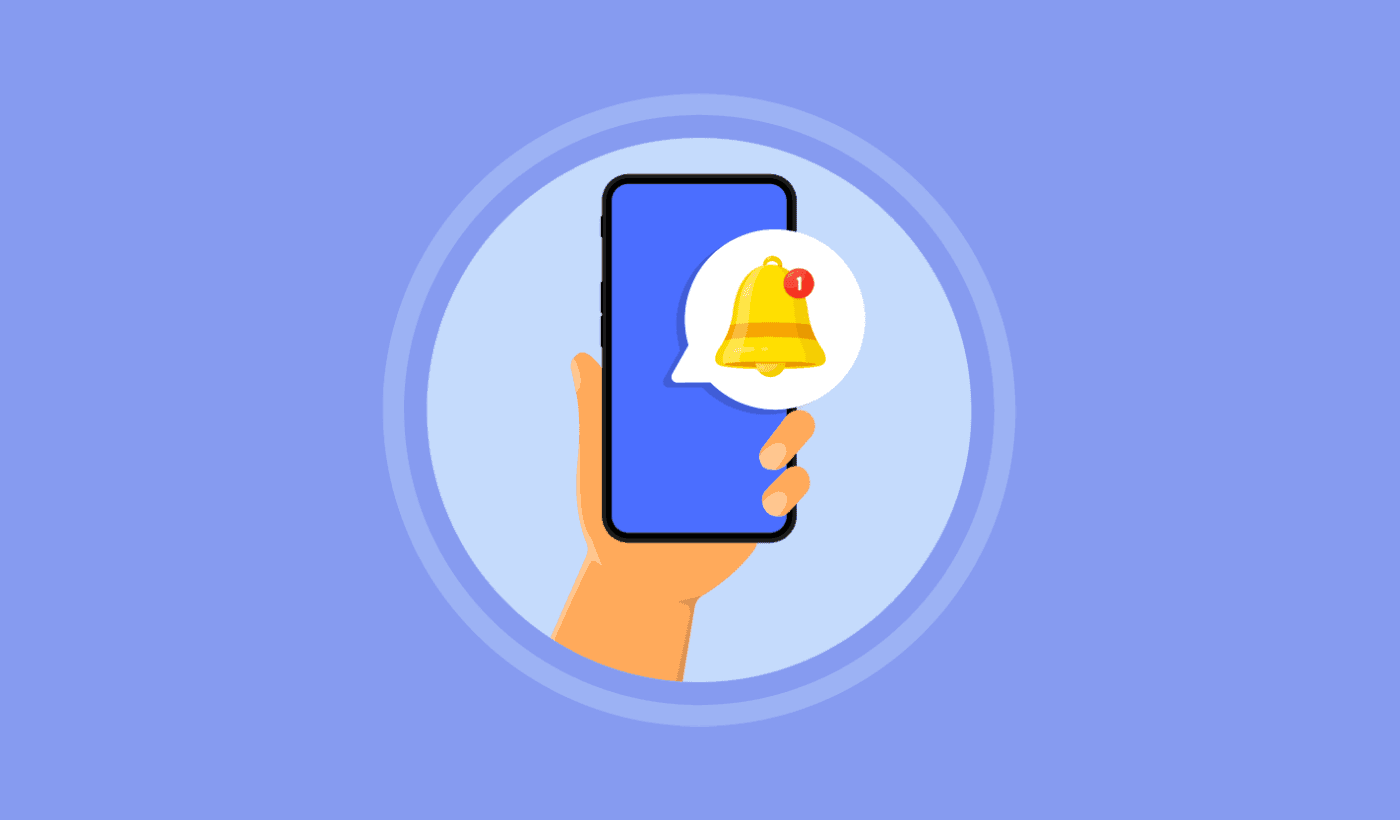
Guide to Set Up Push Notifications in React Native...
1. Create a New React Native Project (if not already created)
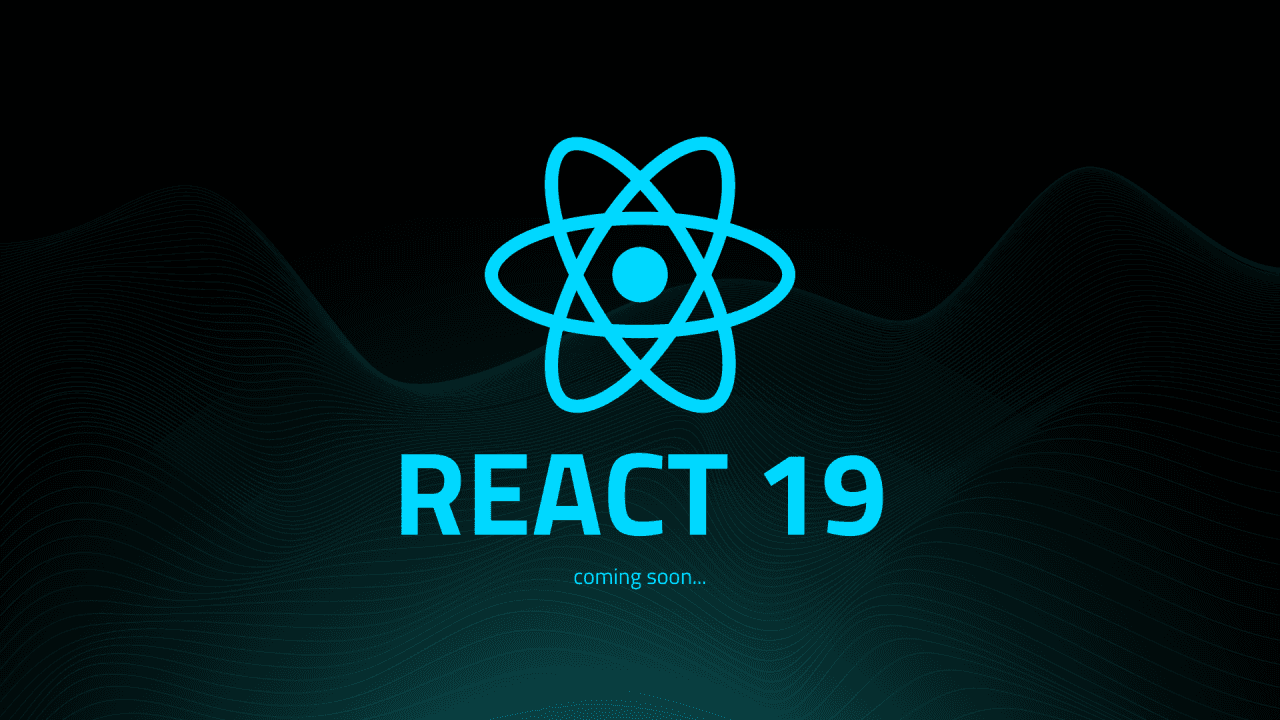
React 19 new features
React version 19 introduces a slew of groundbreaking features aimed at...