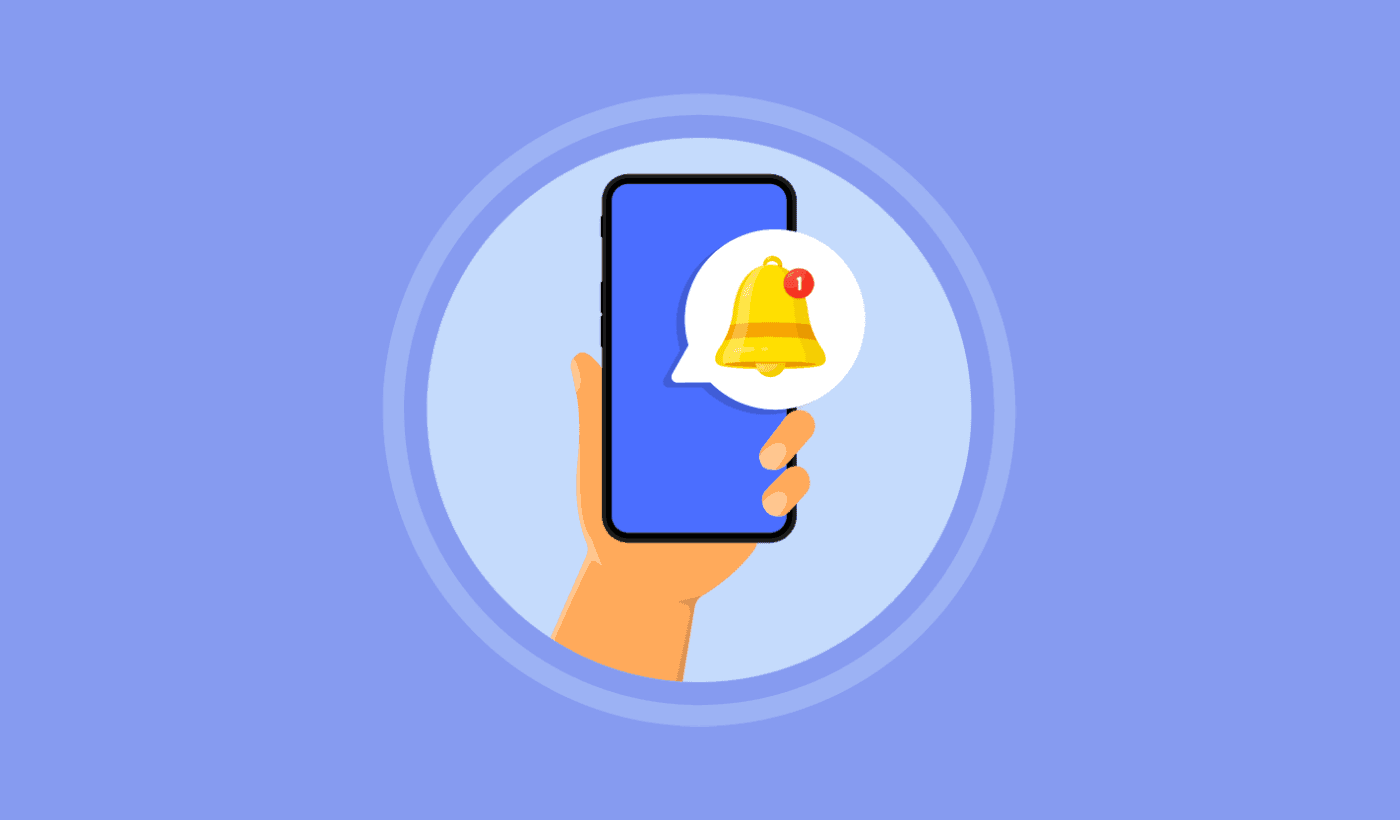
Technology
Guide to Set Up Push Notifications in React Native
By Dwinner ||
10 mins ||
11 December, 2024
1. Create a New React Native Project (if not already created)
If you haven't created a React Native project yet, you can do so with the following command:
npx react-native init PushNotificationsApp
cd PushNotificationsApp
2. Install React Native Firebase
Install react-native-firebase and its dependencies in your project:
npm install @react-native-firebase/app @react-native-firebase/messaging
# or
yarn add @react-native-firebase/app @react-native-firebase/messaging
3. Configure Firebase Project
- Create a Firebase project and add your Android and iOS apps to it in the Firebase console (https://console.firebase.google.com/).
- For Android:
- Download the google-services.json file from Firebase and place it in the android/app directory of your React Native project.
- For iOS:
- Download the GoogleService-Info.plist file from Firebase and add it to the ios/PushNotificationsApp directory of your React Native project.
4. Initialize Firebase in your React Native project
Open your App.js file (or main entry file) and initialize Firebase in your app:
// App.js
import React, { useEffect } from 'react';
import { AppRegistry, Platform } from 'react-native';
import messaging from '@react-native-firebase/messaging';
const App = () => {
useEffect(() => {
// Request permission for notifications
const requestUserPermission = async () => {
const authStatus = await messaging().requestPermission();
const enabled =
authStatus === messaging.AuthorizationStatus.AUTHORIZED ||
authStatus === messaging.AuthorizationStatus.PROVISIONAL;
if (enabled) {
console.log('Authorization status:', authStatus);
}
};
requestUserPermission();
// Handle notifications when app is in foreground
const unsubscribeForeground = messaging().onMessage(async remoteMessage => {
console.log('Received a foreground message:', remoteMessage);
});
return () => {
unsubscribeForeground();
};
}, []);
return (
// Your app's main component
<YourAppMainComponent />
);
};
// Register the app
AppRegistry.registerComponent('PushNotificationsApp', () => App);
export default App;
5. Handle Notifications in the Background
To handle notifications when the app is in the background or terminated, you need to configure a background service handler. This involves modifying your android/app/src/main/java/com/pushnotificationsapp/MainApplication.java file for Android and setting up appropriate handlers as per the Firebase documentation.
6. Testing Push Notifications
- Run your React Native application on an Android or iOS emulator or a physical device.
- Use the Firebase console or a tool like Postman to send test notifications to your device tokens obtained from Firebase Cloud Messaging.
Additional Tips:
- Debugging: Use console logs effectively to debug any issues.
- Handling Notification Data: Extend the code to handle custom data payloads sent with notifications.
- Error Handling: Ensure to handle errors gracefully, especially network-related errors when sending notifications.
This guide provides a basic setup for integrating push notifications in a React Native app using Firebase Cloud Messaging. For production apps, consider additional steps like handling notification clicks, managing notification channels (for Android), and ensuring security best practices.
push-notification
react-native
technology
Featured Post
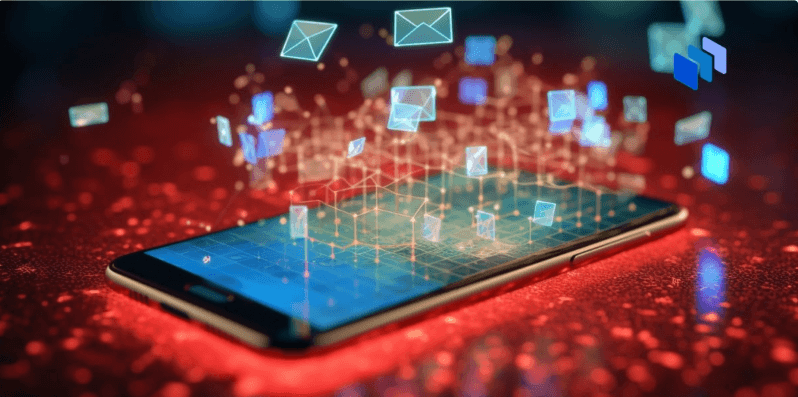
Tech SIM Swap Attacks: Analysis and Protective Mea...
Tech SIM Swap Attacks: Analysis and Protective Measures...
20 June, 2024
10 mins
Recent Post
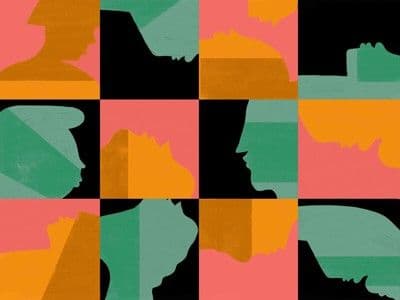
Computer science has a racism problem: these resea...
Black and Hispanic people face huge hurdles at technology companies an...
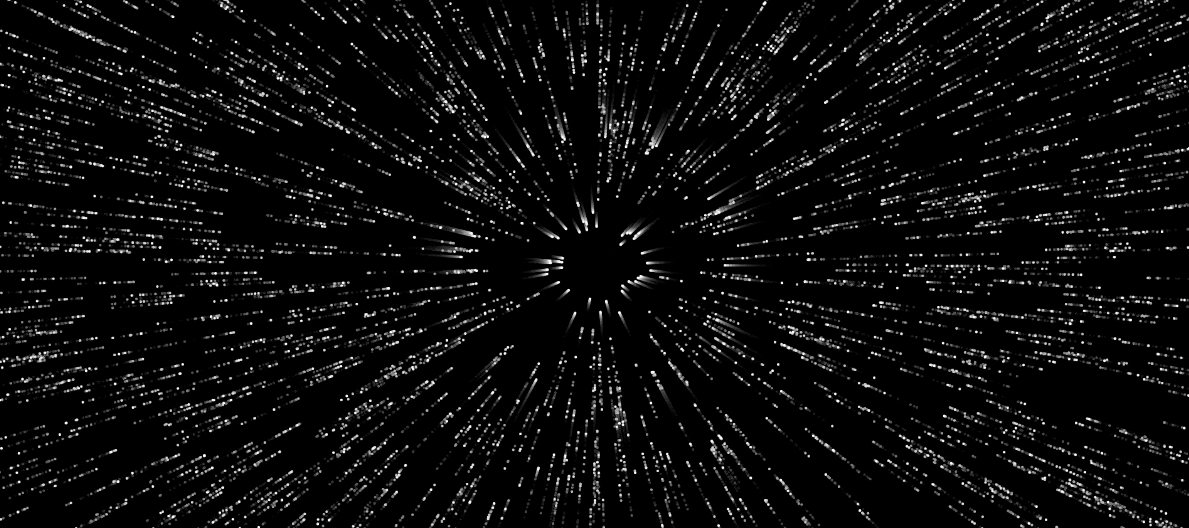
How to Generate Astonishing Animations with ChatGP...
How to Generate Astonishing Animations with ChatGPT
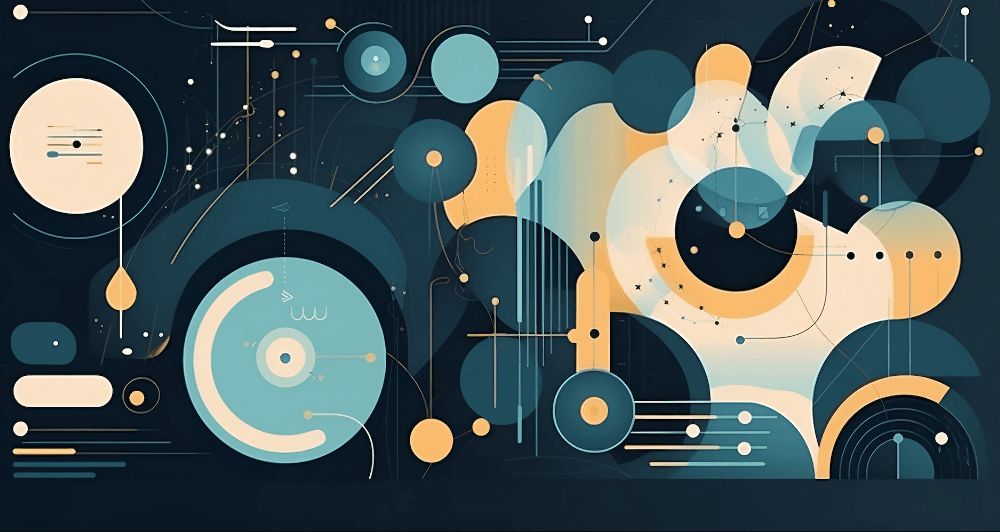
The Web’s Next Transition
The web is made up of technologies that got their start over 25 years ...
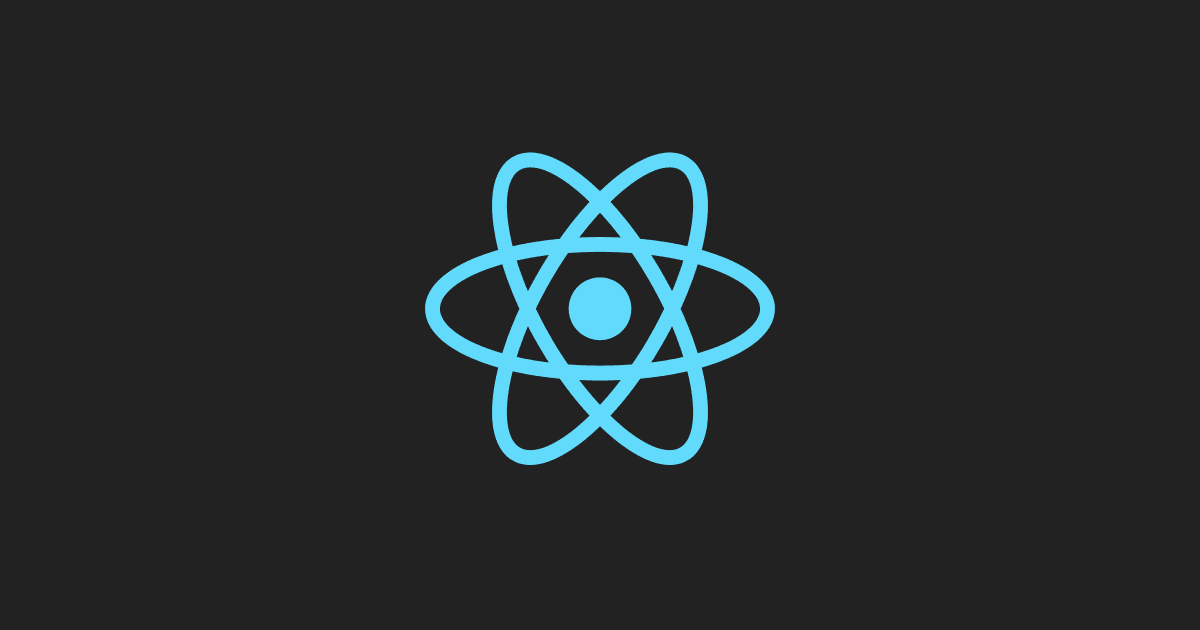
Fundamentals of React Hooks
Hooks have been really gaining a lot of popularity from the first day ...
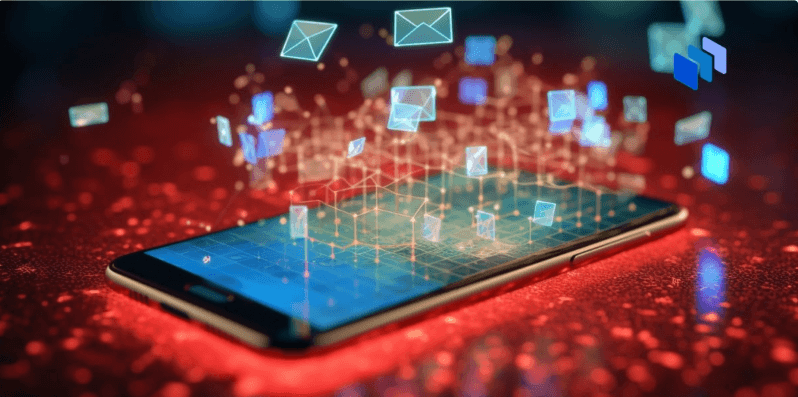
Tech SIM Swap Attacks: Analysis and Protective Mea...
Tech SIM Swap Attacks: Analysis and Protective Measures
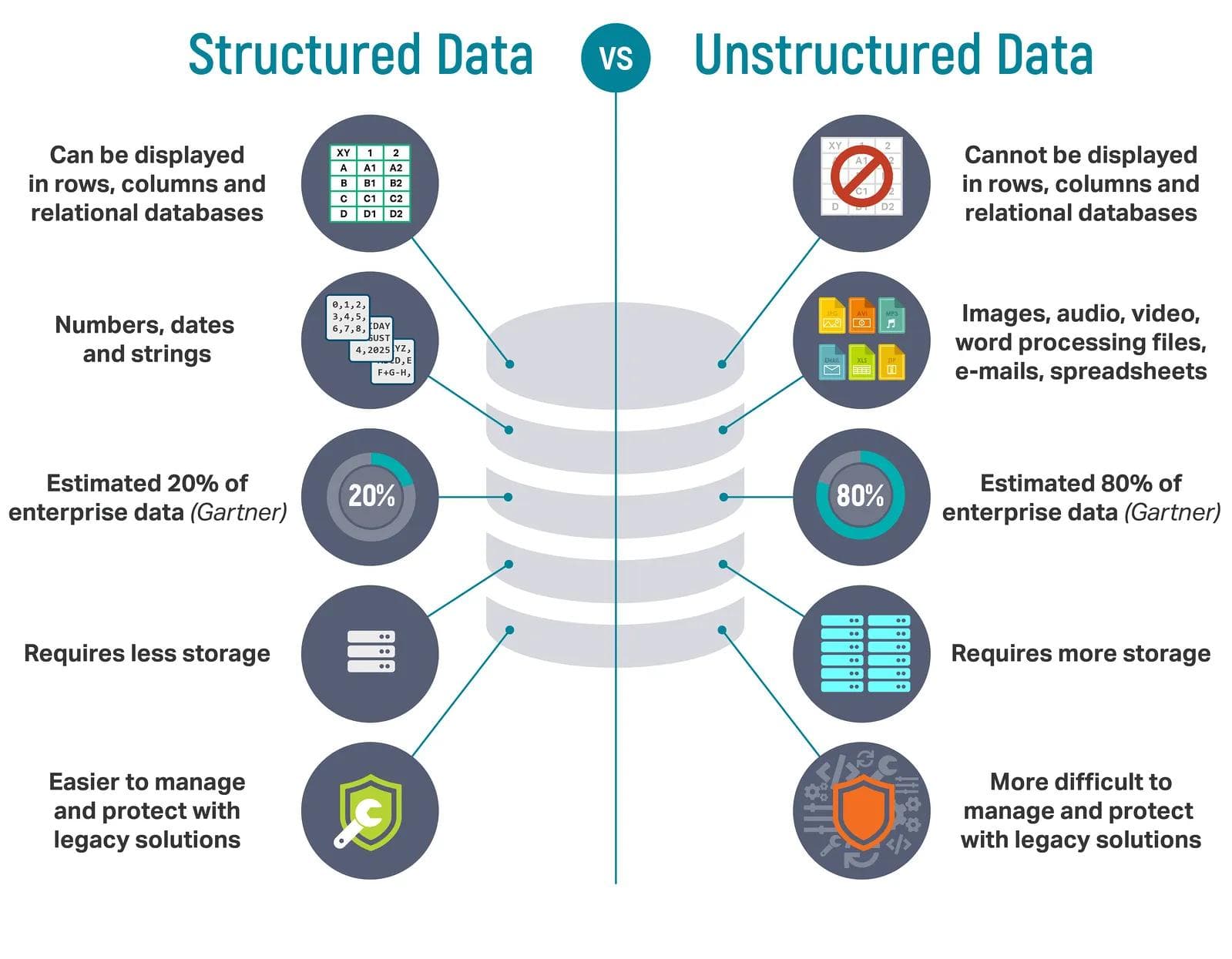
4 Easy Steps to Structure Highly Unstructured Big ...
4 Easy Steps to Structure Highly Unstructured Big Data, via Automated ...
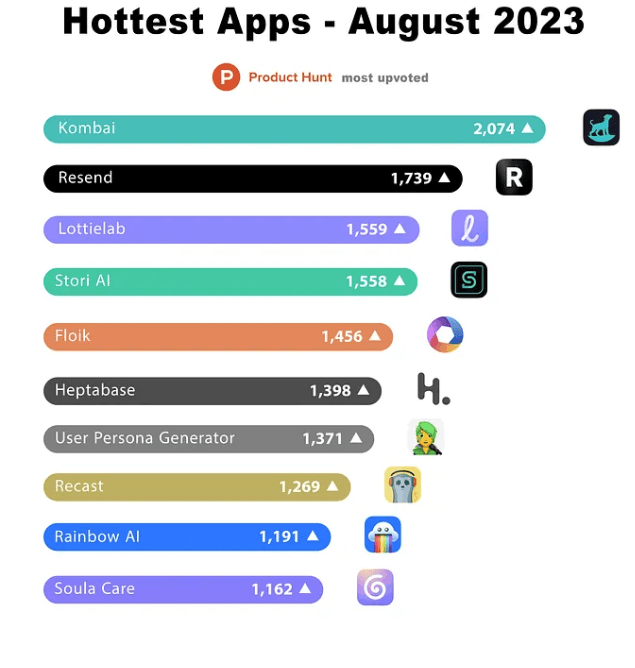
10 Hottest New Apps for You to Get Ahead in Septem...
Here we are again, in a tribute to the amazing stream of ideas that pr...
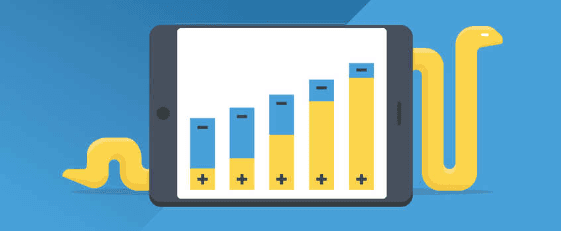
Comparison of Top 6 Python NLP Libraries
Comparison of Top 6 Python NLP Libraries
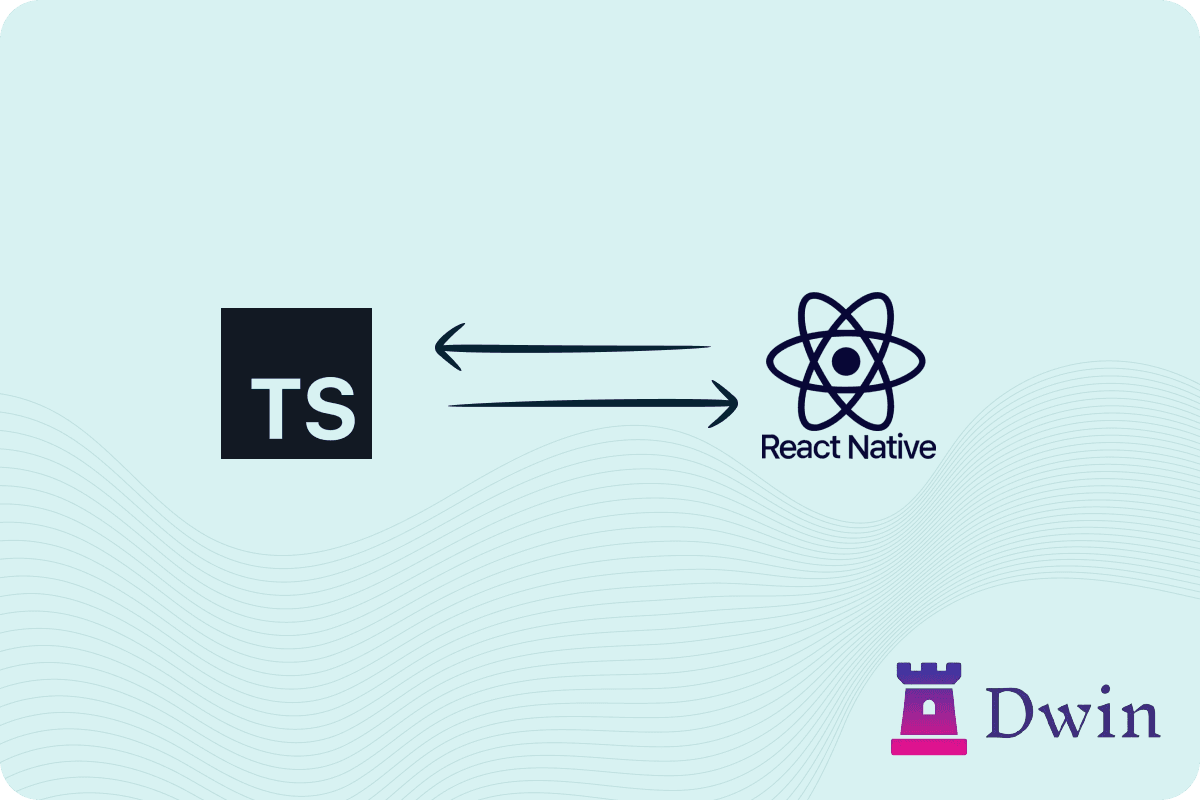
Type Checking with TypeScript in React Native Navi...
📱 In the realm of mobile app development with React Native, ensuring ...
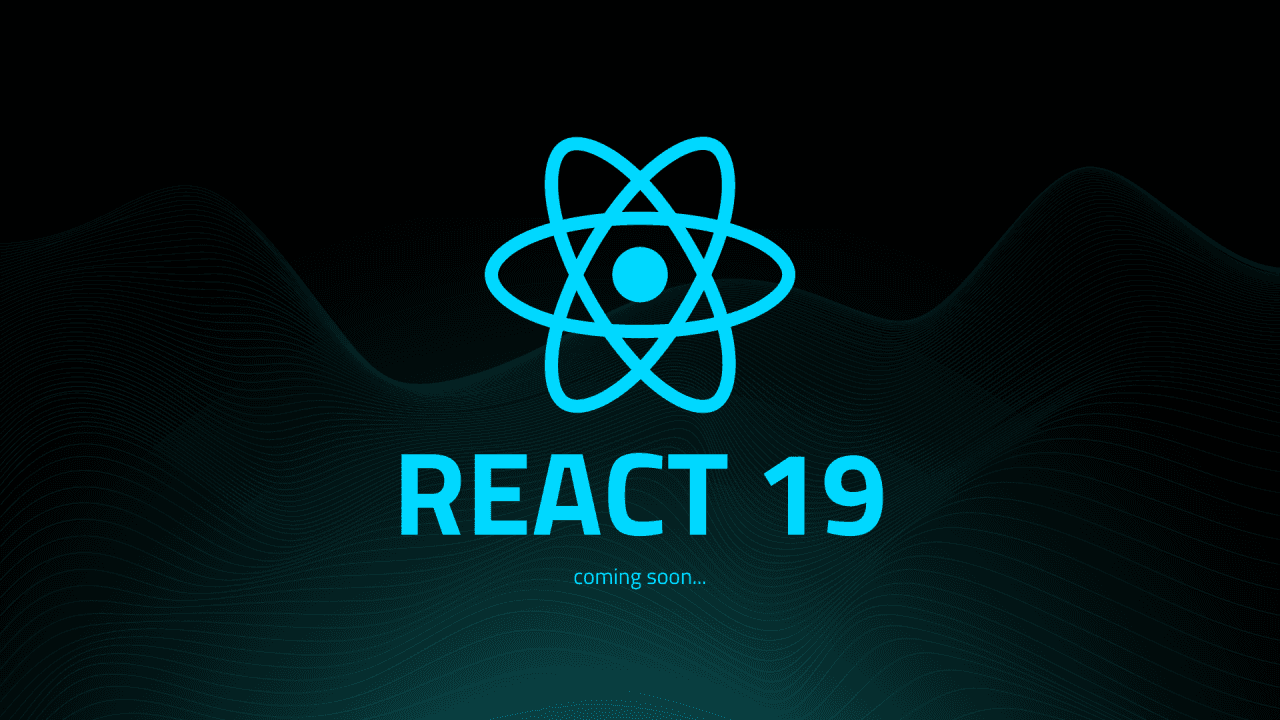
React 19 new features
React version 19 introduces a slew of groundbreaking features aimed at...
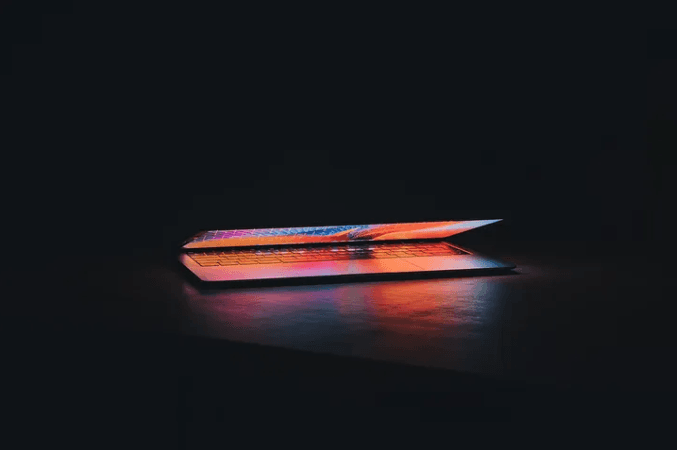
14 amazing macOS tips & tricks to power up your pr...
The mysteriOS Mac-hines keep having more hidden features the more you ...
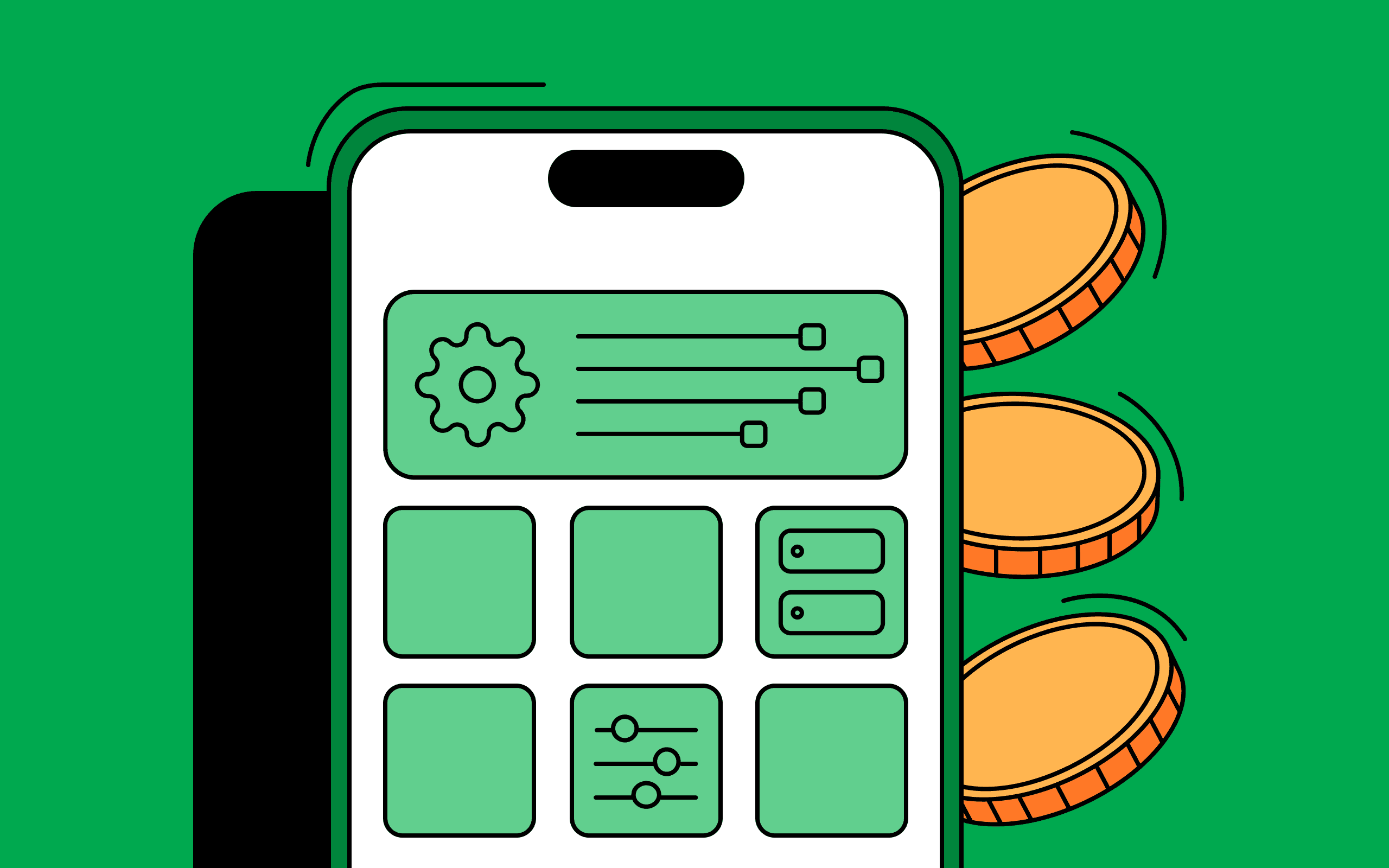
How much does mobile app development cost
The cost of developing a mobile application can vary depending on seve...
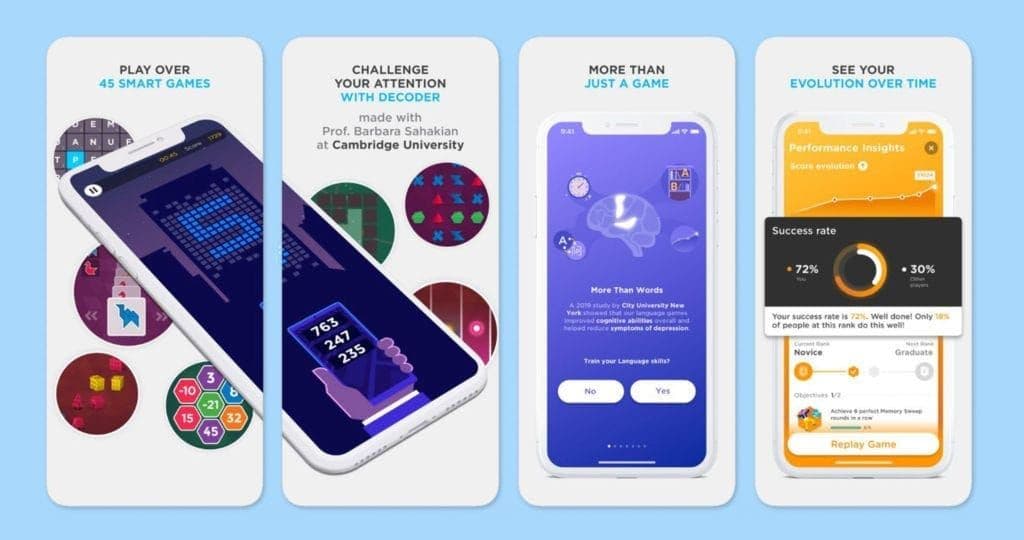
Top 20 mobile apps which nobody knows about…
In the vast ocean of mobile applications, some remarkable gems often g...
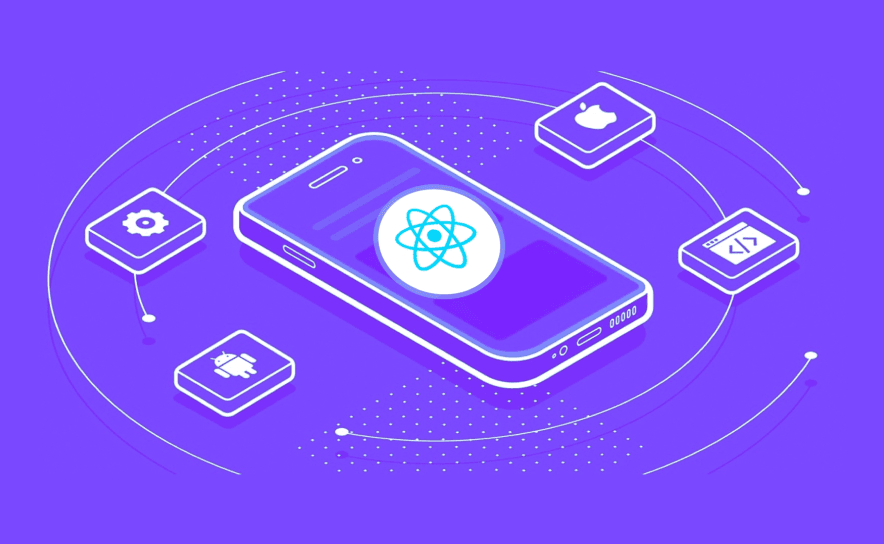
React Native App Development Guide
DWIN, founded in 2015, has swiftly established itself as a leader in c...
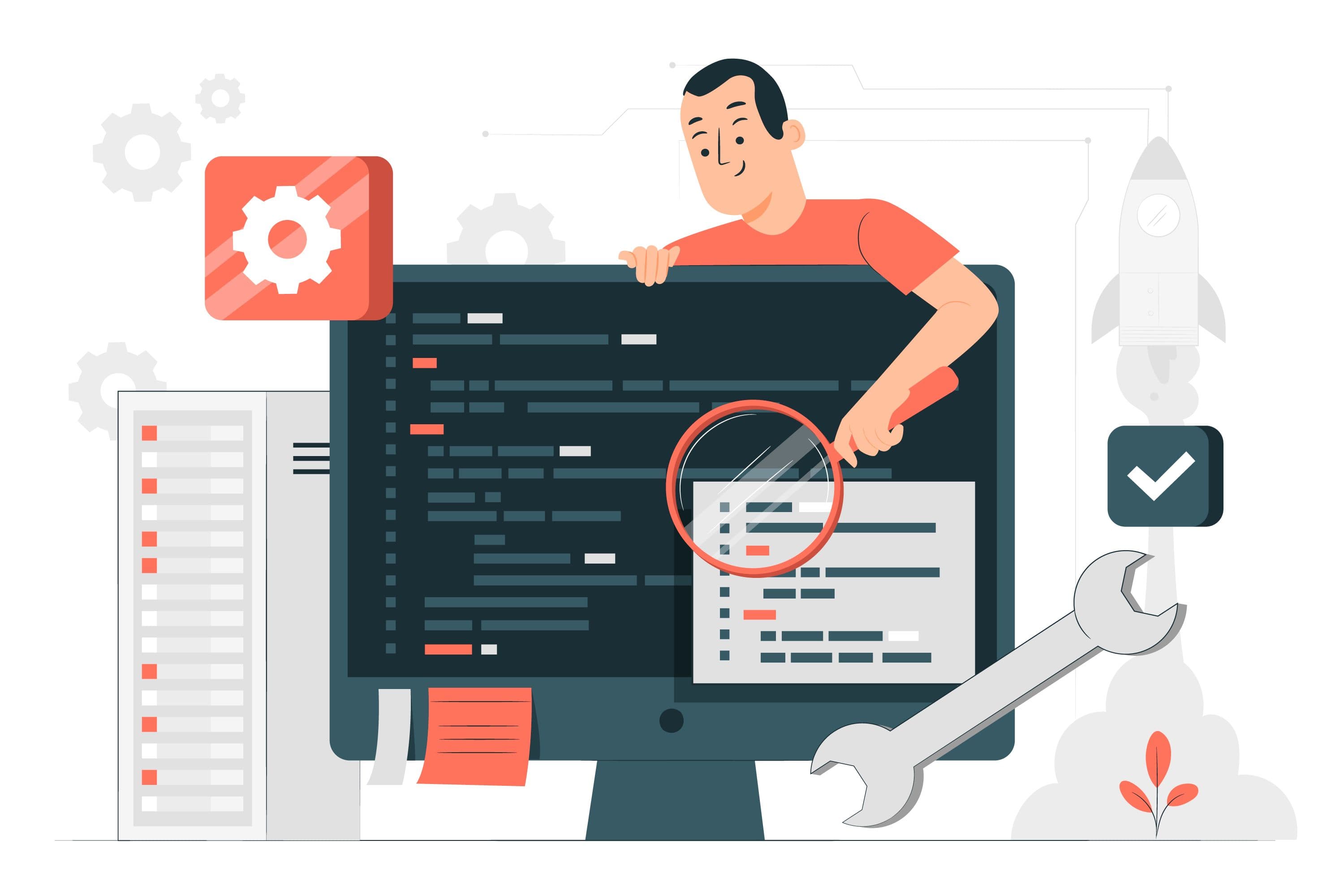
Software Testing: Principles and Stages Simplified...
In the world of software development, testing is not just a phase but ...
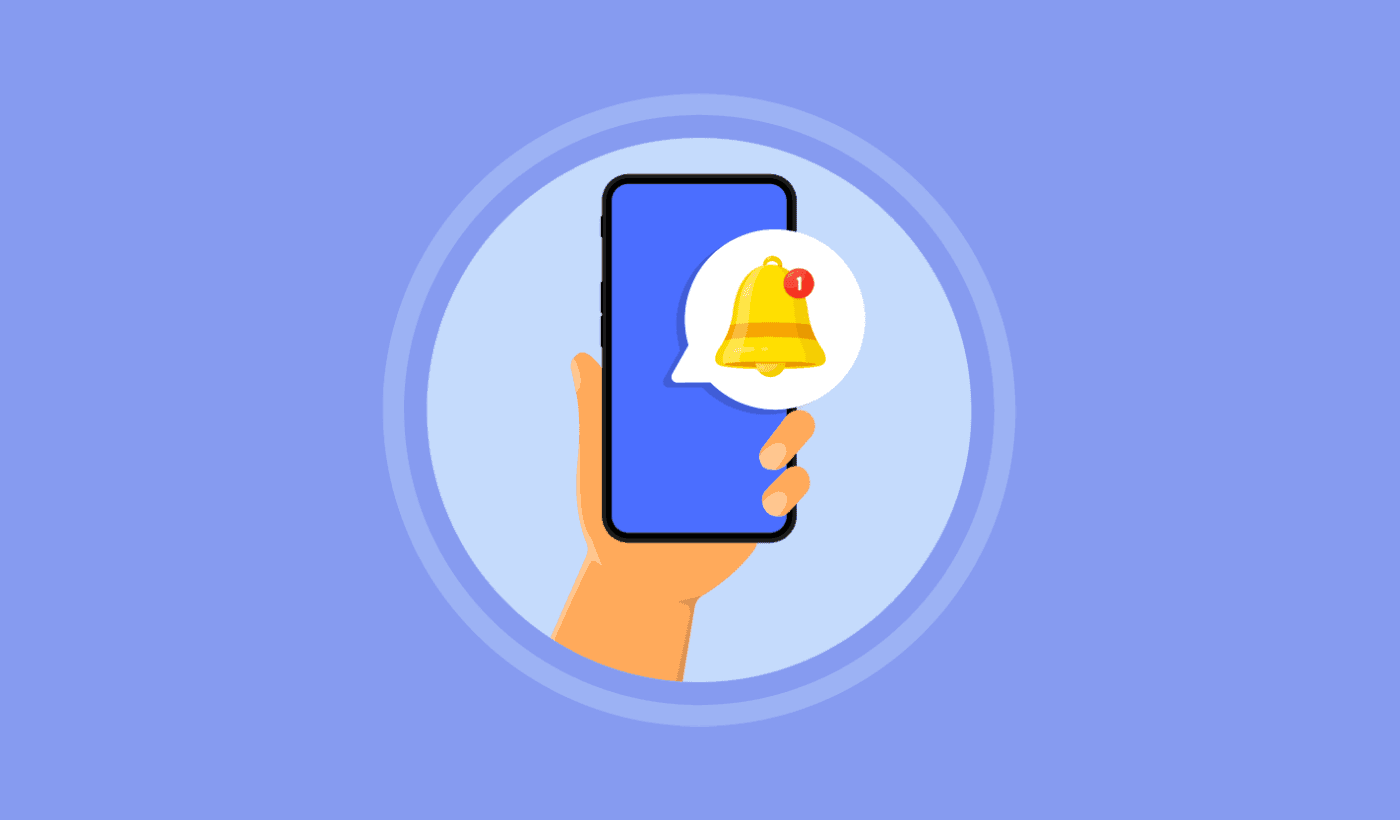
Guide to Set Up Push Notifications in React Native...
1. Create a New React Native Project (if not already created)